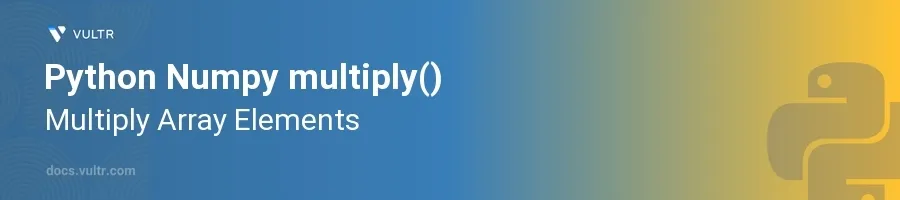
Introduction
The numpy.multiply()
function in Python is a fundamental method for array operations in the NumPy library, which is widely used for numerical computations in Python. It efficiently performs element-wise multiplication across arrays, a crucial operation in many scientific and engineering computations involving matrices and vectors.
In this article, you will learn how to use the numpy.multiply()
function to perform element-wise multiplication of array elements. You will explore examples that demonstrate multiplying scalar values with arrays and the multiplication of two matrices or arrays.
Using multiply() with Scalar Values
Multiply an Array by a Scalar
Import the NumPy library.
Create a NumPy array.
Multiply the array by a scalar value using
numpy.multiply()
.pythonimport numpy as np array = np.array([1, 2, 3, 4]) result = np.multiply(array, 2) print(result)
This code multiplies each element in the array
[1, 2, 3, 4]
by2
, resulting in a new array[2, 4, 6, 8]
.
Use multiply() with Scalar and Multidimensional Array
Define a multidimensional array.
Apply
numpy.multiply()
with a scalar to the array.pythonimport numpy as np matrix = np.array([[1, 2], [3, 4]]) result = np.multiply(matrix, 3) print(result)
Here, each element in the matrix
[[1, 2], [3, 4]]
is multiplied by3
, yielding[[3, 6], [9, 12]]
.
Using multiply() with Arrays
Element-wise Multiplication of Two Arrays
Understand that arrays should be of the same shape for element-wise multiplication.
Create two arrays of the same size.
Multiply the arrays using
numpy.multiply()
.pythonimport numpy as np array1 = np.array([1, 2, 3]) array2 = np.array([4, 5, 6]) result = np.multiply(array1, array2) print(result)
This code multiplies corresponding elements from the two arrays,
[1, 2, 3]
and[4, 5, 6]
, to produce[4, 10, 18]
.
Handling Broadcast in Array Multiplication
Perform Multiplication with Broadcasting
Recognize that NumPy can handle operations on arrays of different sizes using broadcasting.
Use arrays of compatible shapes to demonstrate broadcasting in
numpy.multiply()
.pythonimport numpy as np matrix = np.array([[1, 2], [3, 4]]) vector = np.array([1, 2]) result = np.multiply(matrix, vector) print(result)
This snippet demonstrates broadcasting, where the vector
[1, 2]
is multiplied with each row of the matrix[[1, 2], [3, 4]]
, resulting in[[1, 4], [3, 8]]
.
Conclusion
The numpy.multiply()
function is essential for performing efficient element-wise multiplication of arrays in Python using the NumPy library. Whether you are working with scalars and arrays or two different-sized matrices, numpy.multiply()
supports operations with great flexibility, including handling broadcasting. Utilize this function to manipulate data elements in customized workflows and optimize performance in scientific computing tasks. By following the examples provided, you can effectively incorporate array multiplication into your numerical computing projects, enhancing both the functionality and efficiency of your applications.
No comments yet.