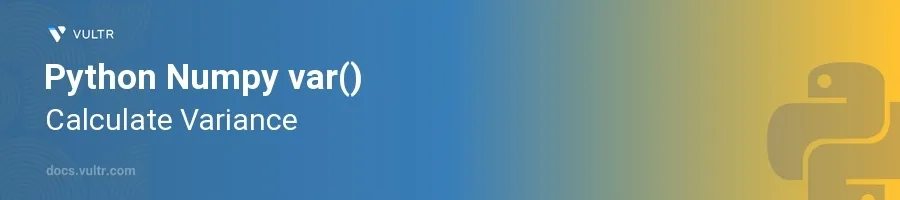
Introduction
In the world of statistics and data analysis, variance is a fundamental measure that quantifies the spread of a set of numbers. In Python, NumPy, a powerful library for numerical operations, offers a straightforward way to compute the variance of data arrays through its var()
function. This function is integral for data scientists and analysts who need to understand the variability or dispersion of their data.
In this article, you will learn how to efficiently use the NumPy var()
function to calculate variance. Discover various applications of this function with different data types and explore how to adjust its behavior using optional parameters to cater to specific analytical needs.
Understanding Variance Calculation
Basic Variance Calculation
Import the NumPy library.
Create an array of data.
Calculate the variance using the
var()
function.pythonimport numpy as np data = np.array([1, 2, 3, 4, 5]) variance = np.var(data) print("Variance:", variance)
This code initializes an array of numbers and computes their variance. The result will encapsulate the average of the squared deviations from the mean, providing a sense of how spread out the numbers are.
Variance of a Multidimensional Array
Handle arrays with more than one dimension.
Use the
axis
parameter to specify the axis along which the variance is computed.pythonmatrix = np.array([[1, 2], [3, 4]]) variance_by_row = np.var(matrix, axis=1) variance_by_column = np.var(matrix, axis=0) print("Variance by Row:", variance_by_row) print("Variance by Column:", variance_by_column)
This snippet demonstrates variance computation across different axes of a matrix. Setting
axis=1
calculates variance across rows, whileaxis=0
addresses columns, offering flexibility depending on data structure needs.
Advanced Usage of var()
Weighted Variance
Adjust calculations for weighted variance where some data points contribute more to the result.
Use the
weights
parameter to specify the weights.pythonweighted_data = np.array([1, 2, 3, 4, 5]) weights = np.array([1, 1, 2, 2, 4]) weighted_variance = np.var(weighted_data, weights=weights) print("Weighted Variance:", weighted_variance)
Applying weights allows for the influence of certain data points to be augmented or diminished, useful in scenarios where data elements have varying importance or reliability.
Handling NaN Values in Data
Understand the pitfalls when dealing with datasets containing NaN (Not a Number) values.
Implement the
where
parameter to specify conditions under which elements are included in the variance calculation.pythondata_with_nan = np.array([1, 2, np.nan, 4, 5]) variance_without_nan = np.var(data_with_nan, where=~np.isnan(data_with_nan)) print("Variance after handling NaN:", variance_without_nan)
By using the
where
parameter, this code effectively excludes NaN values from affecting the variance computation, ensuring a more accurate measure of variability in datasets that might be incomplete or damaged.
Conclusion
The NumPy var()
function is a versatile tool for statistical analysis within Python, providing robust methods to compute variance efficiently across various data types and structures. Whether working with plain number arrays, handling multidimensional data, or managing more complex weighted or incomplete datasets, var()
offers the flexibility and capability needed. Implement the strategies discussed to deepen your analytical abilities and enhance the clarity and precision of your data evaluations.
No comments yet.