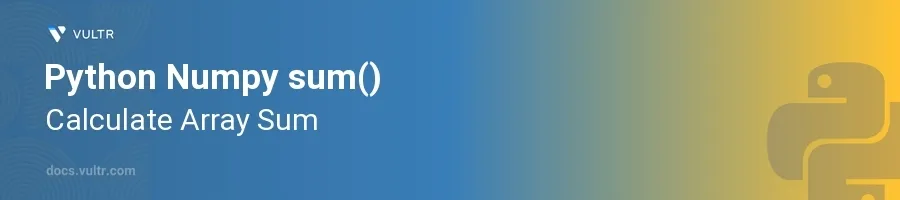
Introduction
The numpy.sum()
function in Python is a vital tool for data analysis, especially when dealing with arrays and matrices. Whether you're summing up elements across different axes of a multidimensional array or calculating the total sum of an array, numpy.sum()
offers a flexible approach. This functionality is critical in tasks ranging from image processing to complex numerical simulations where aggregative summaries of data are required.
In this article, you will learn how to fully utilize the numpy.sum()
function to perform both simple and complex summations. You will explore different scenarios where this function becomes essential including summing specific axes in a multi-dimensional array, handling missing data, and incorporating conditional statements within your summations to refine results.
Understanding the NumPy Sum Function Basics
Calculating the Total Sum of an Array in Python
Start by importing the
numpy
module.Create an array of numbers.
Apply the
sum()
function to compute the total sum.pythonimport numpy as np data = np.array([1, 2, 3, 4]) total_sum = np.sum(data) print(total_sum)
This snippet calculates the sum of all elements in the
data
array, which results in10
. This is a straightforward example wheresum()
processes each element in a one-dimensional array.
Summing Values in Multi-Dimensional Arrays
Consider a multi-dimensional array, such as a 2x3 matrix.
Define the array and then apply
numpy.sum()
without specifying any axis.Observe how it sums all the elements across all dimensions.
pythonarray_2d = np.array([[1, 2, 3], [4, 5, 6]]) sum_all = np.sum(array_2d) print(sum_all)
In this case,
np.sum()
totals every item in the 2-dimensional array to output21
. This example demonstrates the default behavior of summing across all axes.
Specifying Axes for Advanced Summations
Take the same multi-dimensional array and specify an axis with
sum()
.Use axis
0
to sum across the rows (down the columns).Use axis
1
to sum across the columns (across the rows).pythonsum_down_columns = np.sum(array_2d, axis=0) sum_across_rows = np.sum(array_2d, axis=1) print("Sum down the columns: ", sum_down_columns) print("Sum across the rows: ", sum_across_rows)
This code computes the sum of
array_2d
as[5 7 9]
when summed down the columns and[6, 15]
when summed across the rows. Specifying the axis allows targeted summation, useful in many practical scenarios such as statistical analysis across certain dimensions of data.
Handling Missing Data
Summing with NaN Values in the Array
Create an array with
np.nan
values included.Attempt to sum the array with and without handling the NaNs.
pythondata_with_nan = np.array([1, np.nan, 3, 4]) total_with_nan = np.sum(data_with_nan) print("Sum with NaN: ", total_with_nan) # this will typically result in 'nan' total_ignoring_nan = np.nansum(data_with_nan) print("Sum ignoring NaN: ", total_ignoring_nan)
By default,
np.sum()
will returnnan
if any elements arenan
. Usingnp.nansum()
, you can ignore thenan
values and compute the sum of the remaining numbers. This functionality is extremely helpful in datasets with missing entries.
Adding Conditional Logic
Summing with Conditions Using np.where()
Utilize
np.where()
to apply a condition that only numbers greater than a specified value are summed.Combine
np.where()
withnp.sum()
for conditional summation.pythondata = np.array([1, 2, 3, 4, 5]) conditional_sum = np.sum(np.where(data > 2, data, 0)) print("Conditional sum: ", conditional_sum)
This line sums only the elements of
data
that are greater than2
. Thenp.where()
function replaces all other numbers with0
, affecting only specified values in the summation process.
Conclusion
The numpy.sum()
function in Python provides a robust platform for conducting summative analyses on arrays. From handling simple one-dimensional arrays to more complex conditional logic and missing data in multi-dimensional arrays, np.sum()
is both flexible and powerful. Implementing the techniques discussed ensures efficient and accurate data processing. Employing numpy.sum()
helps maintain clarity and performance in numerical computations, making it indispensable for many scientific and analytical applications.
No comments yet.