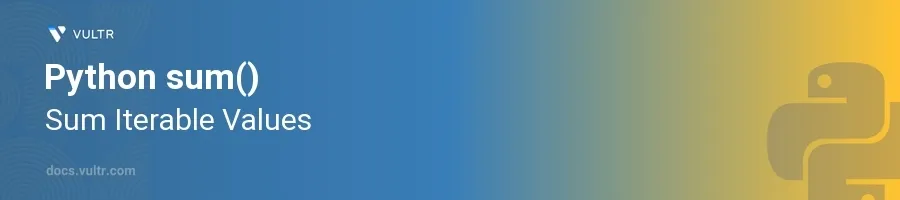
Introduction
The sum()
function in Python provides a straightforward way to calculate the total of the numbers in an iterable, such as a list or tuple. This function is a core part of Python's functionality, allowing programmers to quickly perform arithmetic operations on collections of numerical data without needing to write explicit loops for addition.
In this article, you will learn how to use the sum()
function to sum values in different types of iterables. You will also explore how to apply this function to more complex scenarios, such as when working with lists of lists or when needing to start the summation from a number other than zero.
Basic Usage of sum()
Sum a List of Numbers
Create a list of numeric values.
Use the
sum()
function to calculate the total.pythonnumbers = [10, 20, 30, 40] total = sum(numbers) print(total)
The code above calculates the sum of values in the list
numbers
. The output will be100
as it adds up all the numbers in the list.
Sum a Tuple of Numbers
Define a tuple containing some numbers.
Apply the
sum()
function to get the accumulated result.pythondata = (1, 2, 3, 4) result = sum(data) print(result)
This snippet sums up the numbers in the tuple
data
, resulting in10
.
Advanced Usage of sum()
Sum with a Starting Value
Recognize that
sum()
can accept a second parameter as the starting value.Sum a list starting from a value other than zero.
pythonnumbers = [5, 10, 15] starting_value = 100 total = sum(numbers, starting_value) print(total)
By specifying
100
as the starting value, this function call calculates a total of130
. The summation starts from100
and adds each element from the list.
Sum Nested Lists
For summing nested lists, first flatten the list.
Sum the flattened list using the
sum()
function.pythonnested_lists = [[1, 2], [3, 4], [5, 6]] flattened_list = [item for sublist in nested_lists for item in sublist] total = sum(flattened_list) print(total)
This code first flattens
nested_lists
intoflattened_list
using a list comprehension, then calculates the sum of the flattened list, resulting in21
.
Conclusion
The sum()
function in Python is a versatile and efficient method for aggregating numbers from an iterable. From summing straightforward lists and tuples to working with nested collections and specifying a different starting point, sum()
covers a broad range of needs in numerical data processing. By mastering the various contexts and capabilities of this function, you ensure your code is concise, readable, and efficient in performing summation tasks.
No comments yet.