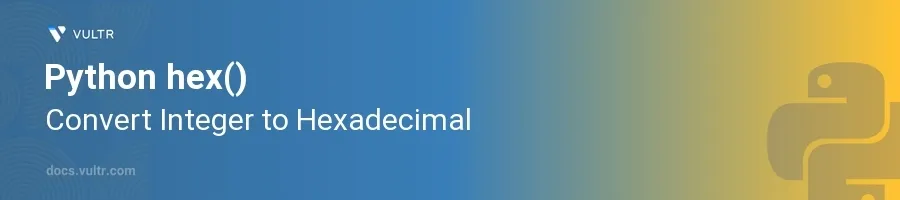
Introduction
The hex()
function in Python is a built-in method used to convert an integer into its corresponding hexadecimal string. This function is particularly useful in fields like cryptography, computer science education, or any scenario where hexadecimal representation is required. Whether you want to convert an integer to hexadecimal in Python for debugging or present an integer in hexadecimal form, the hex()
function provides a quick and reliable solution.
In this article, you will learn how to effectively utilize the hex()
function to convert integers into hexadecimal format. Understand the basic usage of this function, explore its behavior with different types of integers, and see how to format its output for better readability. By the end, you will be equipped to handle various tasks like converting an integer to a hexadecimal string in Python, customizing the output format, and even converting hex numbers back to integers when needed.
Basic Usage of hex() Function in Python
Convert a Positive Integer to Hexadecimal in Python
Define a positive integer.
Use the
hex()
function to convert the integer to a hexadecimal string.pythonnumber = 255 hex_value = hex(number) print(hex_value)
This example converts the integer
255
into its hexadecimal equivalent, which is'0xff'
. This is the easiest method to convert an int to hex in Python.
Convert a Negative Integer to Hexadecimal in Python
Define a negative integer.
Utilize
hex()
to perform the conversion.pythonnumber = -255 hex_value = hex(number) print(hex_value)
Here,
hex()
converts the negative integer-255
to its hexadecimal representation,'-0xff'
. This code will efficiently convert both positive and negative Python int to hex.
Handling Different Integer Types in Python
Convert a Zero to Hexadecimal
Consider the integer zero, which has a special case.
Apply
hex()
for conversion.pythonnumber = 0 hex_value = hex(number) print(hex_value)
In this snippet, the conversion of
0
results in the hexadecimal value'0x0'
. When using Python to convert an integer to a hex string, zero is represented distinctly.
Custom Formatting of Hexadecimal Output
Remove the '0x' Prefix
Convert an integer to hexadecimal.
Slice the string to omit the '0x' prefix.
pythonnumber = 255 hex_value = hex(number)[2:] print(hex_value) # Expected output: 'ff'
This code demonstrates how to display only the hexadecimal digits by removing the '0x' prefix from the string. This is particularly useful when you need to print an integer as a hex string in Python without the 0x prefix.
Format Hexadecimal for Uniform Case
Convert the integer to hexadecimal.
Use string manipulation to ensure all characters are uppercase.
pythonnumber = 255 hex_value = hex(number).upper() print(hex_value) # Expected output: '0XFF'
By calling
.upper()
on the result fromhex()
, you can convert all the hexadecimal characters to uppercase, resulting in'0XFF'
. This is a common requirement when dealing with hexadecimal numbers in Python, where uniformity is essential.
Converting Back from Hexadecimal to Integer in Python
You may sometimes need to reverse the conversion and transform a hexadecimal string back into an integer. This can be achieved using the int()
function with a base of 16
.
Define a hexadecimal string.
Use the
int()
function to convert it to an integer.pythonhex_value = '0xff' number = int(hex_value, 16) print(number) # Output: 255
This method is particularly useful when processing hexadecimal input or decoding hexadecimal representations back to their integer form.
Conclusion
The hex()
function in Python provides a straightforward way to convert integers to their hexadecimal representation, useful for various applications. By mastering this function, you can easily handle common tasks like formatting output or managing numerical data for systems that utilize hexadecimal values. Utilize the techniques discussed to enhance control over the output and ensure your code meets the requirements of any task that involves hexadecimal numbers.
No comments yet.