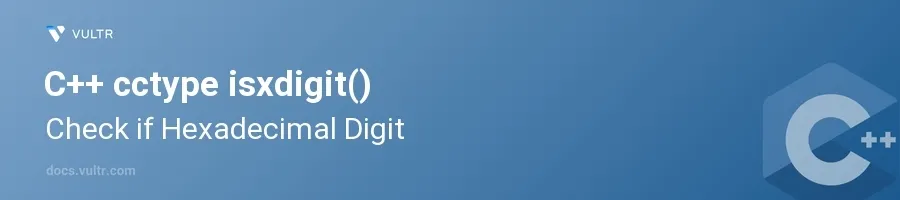
Introduction
The isxdigit()
function in C++ is part of the C++ Character Classification library, housed under the cctype header. It provides a simple and effective method for checking whether a character is a hexadecimal digit, meaning it can test for characters '0'-'9', 'A'-'F', and 'a'-'f'. This functionality is crucial in programming contexts involving data parsing, encoding, or when dealing with hexadecimal values directly, such as in color values or memory addresses.
In this article, you will learn how to utilize the isxdigit()
function to validate and manipulate hexadecimal characters. Explore practical examples to understand how you can implement this function in your C++ applications for more robust data handling and error checking.
Using isxdigit() in C++
Checking Single Characters
Include the
cctype
header in your C++ program to useisxdigit()
.Choose a character and use
isxdigit()
to determine if it is a hexadecimal digit.cpp#include <iostream> #include <cctype> int main() { char ch = 'B'; if (isxdigit(ch)) { std::cout << ch << " is a hexadecimal digit." << std::endl; } else { std::cout << ch << " is not a hexadecimal digit." << std::endl; } return 0; }
This program checks if the character
ch
is a hexadecimal digit. Since 'B' falls within the range of valid hexadecimal digits ('A' to 'F'), it output that it is a hexadecimal digit.
Evaluating Strings
Iterate over each character in a string.
Check each character with
isxdigit()
to verify the string's validity as a hexadecimal number.cpp#include <iostream> #include <cctype> #include <string> bool isHexadecimal(const std::string &str) { for (char c : str) { if (!isxdigit(c)) { return false; } } return true; } int main() { std::string hexStr = "1A3F"; if (isHexadecimal(hexStr)) { std::cout << hexStr << " is a valid hexadecimal number." << std::endl; } else { std::cout << hexStr << " is not a valid hexadecimal number." << std::endl; } return 0; }
In this example, the function
isHexadecimal()
checks if every character inhexStr
is a hexadecimal digit. The string "1A3F" is composed entirely of valid hexadecimal characters, so the output confirms its validity.
Conclusion
The isxdigit()
function in C++ provides a straightforward way to validate whether a character or a set of characters form valid hexadecimal digits. It is a powerful tool for ensuring data integrity and correctness, particularly when dealing with systems that process or display hexadecimal values frequently. By deploying the examples presented, maintain high data validation standards in your C++ programs, ensuring your applications handle hexadecimal data efficiently and correctly.
No comments yet.