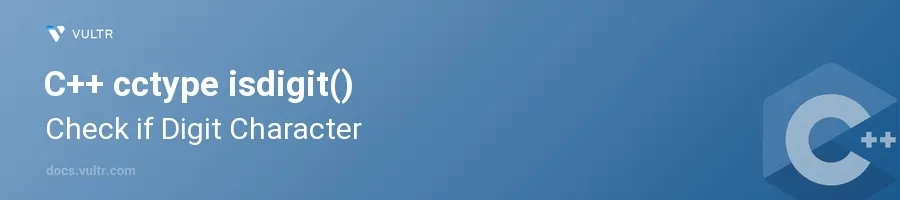
Introduction
The isdigit()
function from the C++ cctype
library provides a straightforward way to check if a character represents a numeric value between '0' and '9'. This function is highly useful when your code needs to validate user input or process strings that contain different types of characters.
In this article, you will learn how to use the isdigit()
function effectively in various scenarios. Understand how to incorporate it into C++ programs for checking single characters as well as strings for numeric characters. Explore its behavior with different character inputs to ensure robust data handling in your applications.
Using isdigit() to Evaluate Characters
Check if a Single Character is a Digit
Include the
<cctype>
library in your C++ program to accessisdigit()
.Initialize a character variable to be checked.
Use
isdigit()
to determine if the character is a digit.cpp#include <cctype> #include <iostream> char ch = '5'; bool isDigit = isdigit(ch); std::cout << "Is digit: " << isDigit << std::endl;
This code checks if the character
ch
is a digit. As '5' is a numeric value,isdigit()
will return a truthy value indicatingtrue
.
Handling Non-Digit Characters
Test
isdigit()
with various non-digit inputs to see how it behaves.Initialize a character which is not a digit.
Apply
isdigit()
and display the result.cppchar ch = 'a'; bool isDigit = isdigit(ch); std::cout << "Is digit: " << isDigit << std::endl;
In this example, since
a
is not a numeric character,isdigit()
returns a falsy value, which is printed as0
(false).
Use Cases of isdigit() in String Processing
Verifying All Characters in a String are Digits
Create a string comprised of several characters.
Iterate over each character in the string and use
isdigit()
to check each one.Return a result based on the checks.
cpp#include <cctype> #include <iostream> #include <string> std::string str = "12345"; bool allDigits = true; for(char& c : str) { if (!isdigit(c)) { allDigits = false; break; } } std::cout << "All characters are digits: " << allDigits << std::endl;
This code determines if all characters in
str
are digits. It iterates through the string, checking each character withisdigit()
. Since all characters are digits, the result istrue
.
Identifying Digit Characters in Mixed Strings
Employ
isdigit()
to find and print all numeric characters in a mixed content string.Loop through the string and check each character.
cppstd::string str = "Hello 2023!"; std::cout << "Digits in the string: "; for(char& c : str) { if (isdigit(c)) { std::cout << c << " "; } } std::cout << std::endl;
In this snippet,
isdigit()
is used to selectively print only those characters from the stringstr
that are numeric, providing a clear view of all digits in a mixed string.
Conclusion
Utilizing the isdigit()
function from the C++ cctype
library helps you ensure that the characters or strings your program processes meet specific numeric criteria. Whether checking individual characters or validating entire strings, isdigit()
offers a reliable method for detecting numeric characters. Implement these techniques in your projects to handle data validation and string processing tasks efficiently with precision.
No comments yet.