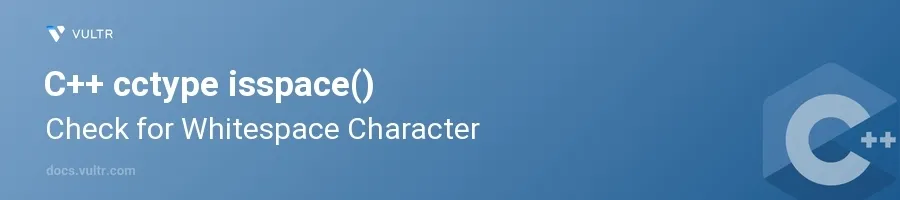
Introduction
The isspace()
function in C++ is a standard library function that checks if a given character is a whitespace character. Whitespace characters typically include spaces, tabs, new line characters, and other similar types. This function is part of the <cctype>
header and is widely used in parsing and processing text where determining the segmentation of words or lines based on spaces is crucial.
In this article, you will learn how to properly use the C++ isspace()
function to detect whitespace characters in different contexts. Explore how to integrate this function in text processing tasks such as counting whitespace, filtering strings, and validating user input.
Using isspace() Function
Setting Up the Environment
Before using the isspace()
function, ensure you have included the <cctype>
header in your C++ program.
#include <cctype>
Including this header file gives you access to various character classification functions including isspace()
.
Checking for Whitespace Characters
Define a character variable.
Use
isspace()
to check if this character is a whitespace.cppchar testChar = ' '; bool isSpace = isspace(testChar);
In this code,
isspace(testChar)
returns a non-zero value (true) sincetestChar
is a space character, which is a type of whitespace.
Handling Different Types of Whitespace
Use multiple characters including tabs and new lines.
Verify that
isspace()
correctly identifies each.cppchar space = ' '; char tab = '\t'; char newline = '\n'; bool spaceCheck = isspace(space); bool tabCheck = isspace(tab); bool newlineCheck = isspace(newline);
This will correctly identify space, tab, and newline as whitespace characters, showing the utility of
isspace()
in handling various types of whitespace.
Using isspace() in a String
Loop through each character in a string.
Use
isspace()
to test each character for whitespace.cpp#include <iostream> #include <cstring> using namespace std; int main() { char str[] = "Hello World\t\n"; int count = 0; for (int i = 0; i < strlen(str); ++i) { if (isspace(str[i])) { count++; } } cout << "Number of whitespace characters: " << count << endl; return 0; }
This program counts how many times whitespace characters appear in the string
str
, showcasing the practical usage ofisspace()
in real-world applications like text processing.
Conclusion
The isspace()
function in C++ provides a simple yet powerful way to identify whitespace characters in strings. By leveraging this function, you can handle common tasks such as validating text input, parsing text data, or counting spaces. Use the examples discussed to incorporate isspace()
effectively in your C++ projects to enhance text analysis and manipulation capabilities. Remember, recognizing and effectively using character classification functions forms the foundation of efficient and effective text processing.
No comments yet.