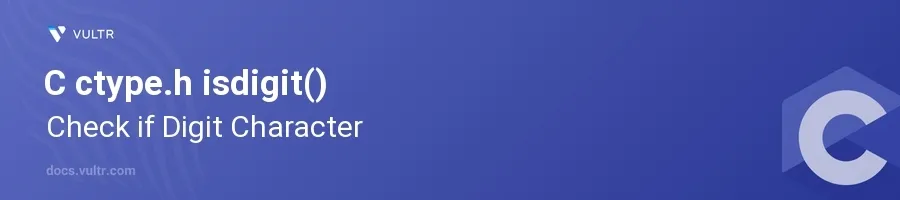
Introduction
The isdigit()
function from the C standard library's ctype.h
header file is a simple yet powerful utility for checking if a character is a numeric digit. This function is particularly useful when parsing strings to extract numbers, validating user input, or processing text data that includes numerical characters.
In this article, you will learn how to leverage the isdigit()
function in your C programs. Explore how this function works, understand its implementation details, and see practical examples of it in action.
Understanding isdigit()
Basic Usage of isdigit()
Include the
ctype.h
header file in your C program to accessisdigit()
.Pass the character you want to check as an argument to the function.
c#include <stdio.h> #include <ctype.h> int main() { char c = '5'; if (isdigit(c)) { printf("%c is a digit.\n", c); } else { printf("%c is not a digit.\n", c); } return 0; }
In this example,
isdigit(c)
checks ifc
is between '0' and '9'. As '5' is a digit, it prints that '5' is a digit.
Character Encoding Consideration
Recognize that
isdigit()
works correctly with standard ASCII and extended ASCII values.Ensure the character being tested is unsigned or cast to unsigned char to avoid undefined behavior.
c#include <stdio.h> #include <ctype.h> int check_digit(int c) { return isdigit((unsigned char)c); } int main() { char test_char = '8'; // ASCII for '8' is 56 printf("%c is %s a digit.\n", test_char, check_digit(test_char) ? "" : "not"); return 0; }
Casting the character to
unsigned char
ensures that theisdigit()
function exhibits defined behavior even if the input is a signed character with a negative value.
Practical Applications of isdigit()
Validating Numeric Input
Use
isdigit()
to validate if a string consists exclusively of digit characters.c#include <stdio.h> #include <ctype.h> int is_numeric_string(const char *str) { while (*str) { if (!isdigit((unsigned char)*str)) { return 0; // Non-digit character found } str++; } return 1; // All characters were digits } int main() { char num_str[] = "12345"; if (is_numeric_string(num_str)) { printf("The string is numeric.\n"); } else { printf("The string is not numeric.\n"); } return 0; }
This function iterates over each character of the string, checking if each is a digit. It returns
1
if all characters are digits, otherwise0
.
Parsing Numbers from Strings
Combine
isdigit()
with other C functions to extract numbers from a mixed string.c#include <stdio.h> #include <ctype.h> int main() { char str[] = "Item 24 count 36"; int numbers[10]; int i = 0, num = 0; for (char *p = str; *p; p++) { if (isdigit((unsigned char)*p)) { num = num * 10 + (*p - '0'); if (!isdigit((unsigned char)*(p + 1))) { numbers[i++] = num; num = 0; } } } printf("Extracted numbers: "); for (int j = 0; j < i; j++) { printf("%d ", numbers[j]); } printf("\n"); return 0; }
This code reads characters sequentially and accumulates a number until a non-digit character is encountered, then resets and starts accumulating the next number.
Conclusion
The isdigit()
function in C is essential for processing numerical data within text, validating inputs, and parsing numbers from strings. Its straightforward usage and clear functionality make it an indispensable tool in any C programmer's toolkit. By understanding and implementing the techniques discussed, you enable your applications to handle numeric character data effectively and efficiently.
No comments yet.