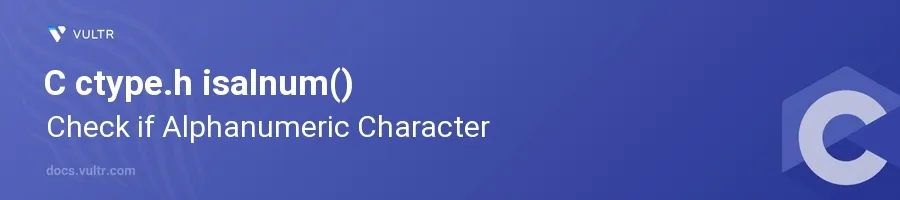
Introduction
The isalnum()
function in C, provided by the ctype.h
library, checks whether a given character is alphanumeric, combining both alphabetic (letters) and numeric (digits) characters. This function proves to be extremely valuable when parsing strings or validating inputs to ensure data integrity in various applications ranging from simple user input forms to complex data parsers.
In this article, you will learn how to effectively deploy the isalnum()
function in your C programs. Explore the scenarios for using isalnum()
in single character evaluations, string validations, and highlight how it integrates within common programming tasks.
Understanding isalnum() Function
Basic Usage of isalnum()
Include the
ctype.h
library in your program.Initialize a character variable.
Use
isalnum()
to check if the character is alphanumeric.c#include <ctype.h> #include <stdio.h> int main() { char c = 'a'; if (isalnum(c)) { printf("%c is alphanumeric\n", c); } else { printf("%c is not alphanumeric\n", c); } return 0; }
This code snippet includes the necessary library and employs
isalnum()
to verify if the character stored inc
is alphanumeric. The result indicates that 'a' is, indeed, alphanumeric.
Verifying Strings for Alphanumeric Characters
Read or define a string.
Loop over each character in the string.
Apply the
isalnum()
function to each character to check its nature.c#include <ctype.h> #include <stdio.h> int main() { char str[] = "Hello123"; int alphaNum = 1; // Assume the string is alphanumeric for (int i = 0; str[i] != '\0'; i++) { if (!isalnum(str[i])) { alphaNum = 0; // Set to false if any character is not alphanumeric break; } } if (alphaNum) { printf("The string is alphanumeric.\n"); } else { printf("The string has non-alphanumeric characters.\n"); } return 0; }
The code iterates over each character in the string
str
and usesisalnum()
to validate. If all characters are alphanumeric, the loop completes withalphaNum
remaining true; otherwise, it setsalphaNum
to false upon encountering any non-alphanumeric character.
Applying isalnum() in Conditional Statements
Implement Efficient Data Validation
Define a function to validate user IDs or data entries.
Utilize
isalnum()
to ensure these entries contain only alphanumeric characters.c#include <ctype.h> #include <stdio.h> #include <string.h> int validateUserID(char *userID) { for (int i = 0; i < strlen(userID); i++) { if (!isalnum(userID[i])) { return 0; // Invalid user ID } } return 1; // Valid user ID } int main() { char userID[] = "JohnDoe123"; if (validateUserID(userID)) { printf("User ID is valid\n"); } else { printf("User ID is invalid\n"); } return 0; }
This function checks every character in the
userID
string. If any character is not alphanumeric, it returns false, indicating an invalid user ID. Otherwise, it confirms the user ID as valid.
Conclusion
By mastering the isalnum()
function from the C ctype.h
library, you ensure your program can effectively evaluate the nature of characters and strings for alphanumeric content. Whether checking individual characters, validating entire strings, or securing user inputs, this function is indispensable for maintaining data integrity and effective input validation. With the provided examples and usage scenarios, you now have the tools to implement this functionality in various C programming contexts, enhancing both the security and robustness of your applications.
No comments yet.