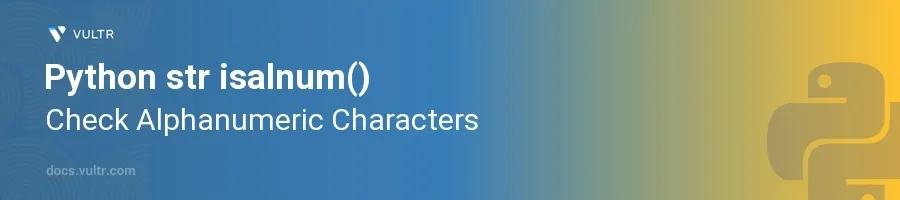
Introduction
The isalnum()
method in Python strings is a straightforward tool for validating whether the content of a string consists exclusively of alphanumeric characters (letters and numbers). This method is particularly useful in situations where data needs to be sanitized or validated before processing, such as when handling user input or parsing text files.
In this article, you will learn how to use the isalnum()
method effectively. Explore how this method can be applied to check the composition of strings and ensure they meet specific criteria necessary for further processing or data validation.
Using str isalnum() to Check for Alphanumeric Characters
Basic Usage of isalnum()
Define a string that you want to check.
Apply
isalnum()
directly to the string and store or display the result.pythonsample_text = "Python3" result = sample_text.isalnum() print(result)
This code snippet checks the string
sample_text
to determine if all characters are alphanumeric. The expected output isTrue
since "Python3" includes letters and a digit, all of which are alphanumeric.
Handling Strings with Spaces and Special Characters
Recognize that spaces, punctuation, and symbols return
False
withisalnum()
.Define a string containing spaces or non-alphanumeric characters.
Use
isalnum()
and display the result.pythonsample_text = "Python 3.8" result = sample_text.isalnum() print(result)
In this example, the output is
False
because the space and the period are not alphanumeric characters.isalnum()
efficiently identifies cases where non-valid characters are involved.
Practical Example: Validating User Input
Imagine a use case where it's important to ensure a username consists only of alphanumeric characters.
Prompt the user for input and apply
isalnum()
to validate it.pythonusername = input("Enter your username: ") if username.isalnum(): print("Username is valid!") else: print("Username can only contain letters and numbers.")
This block efficiently checks if the entered
username
is valid according to set criteria – being strictly alphanumeric. It improves data integrity in applications where usernames are involved.
Conclusion
The isalnum()
function is a valuable tool for checking if a string contains only alphanumeric characters, without including spaces, symbols, or punctuation. This method proves particularly useful in validating user inputs and ensuring data purity in text processing applications. By integrating the examples demonstrated, you enhance your system's ability to handle and verify string data effectively, thus boosting the robustness and security of your programs.
No comments yet.