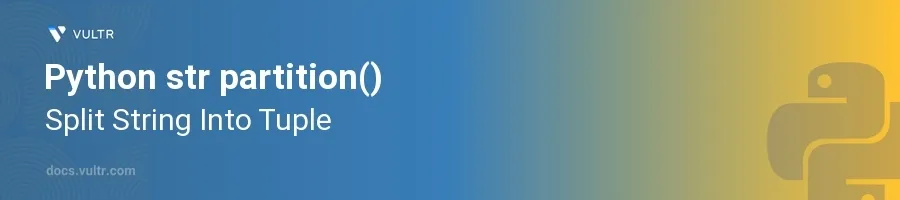
Introduction
The partition()
method in Python is a string operation that splits a specified string into a tuple containing three elements. This method takes a string argument as a "separator" and divides the string into parts before the separator, the separator itself, and the remaining text after the separator. It serves as a valuable tool when you need to dissect a string based on the presence of a specific substring, ensuring that both the separator and surrounding content are captured accurately.
In this article, you will learn how to employ the partition()
method in practical string manipulation scenarios. Explore useful examples demonstrating when and how to utilize this method to efficiently manage and analyze string data, including handling cases where the separator is not found in the string.
Understanding Python's str.partition()
Basics of str.partition()
The partition()
method searches for the given separator and returns a tuple: the first element is the part of the string before the separator, the second is the separator itself, and the third is the part of the string after the separator.
Start by defining a string.
Choose a separator to partition the string.
Apply the
partition()
method.pythonsentence = "Python is powerful and fast" separator = "is" result = sentence.partition(separator) print(result)
This code splits the sentence into a tuple
('Python ', 'is', ' powerful and fast')
. The output contains the texts surrounding 'is' and the separator itself.
Handling Non-Existent Separators
When the specified separator is not present in the string, the partition()
method returns a tuple: the original string, followed by two empty strings.
Choose a string and a separator not present in the string.
Use the
partition()
method.pythonsentence = "Hello, world!" separator = "easy" result = sentence.partition(separator) print(result)
Here, since "easy" does not exist in the "Hello, world!" string, the output will be
('Hello, world!', '', '')
, with the original string intact and two empty strings following it.
Multiple Occurrences of the Separator
The partition()
method only partitions the string at the first occurrence of the separator. It's useful for situations where only the first instance of a separator is relevant.
Define a string with multiple instances of a separator.
Use
partition()
to split the string.pythoninfo = "Name: John; Age: 30; Name: Jane; Age: 25" separator = ";" result = info.partition(separator) print(result)
The output will be
('Name: John', ';', ' Age: 30; Name: Jane; Age: 25')
, showing how only the first occurrence of";"
affected the split.
Practical Applications of str.partition()
Extracting Key-Value Pairs
The partition()
method is excellent for extracting key-value pairs in configurations or simple data strings where entries are separated by a unique character.
Consider a string formatted as key-value pairs.
Split the string and process each piece.
pythondata = "username=johndoe" key, separator, value = data.partition("=") print(f"Key: {key}, Value: {value}")
In this scenario, the
partition()
method helps separate the key and the value efficiently, giving a clear output ofKey: username, Value: johndoe
.
Parsing URLs or Paths
When working with URLs or file paths, partition()
can isolate parts of the string for further processing or validation.
Take a URL or path string.
Use
partition()
to separate the protocol or directory.pythonurl = "http://example.com/page" protocol, separator, path = url.partition("://") print(f"Protocol: {protocol}, Path: {path}")
This demonstrates splitting a URL into its protocol and path components, simplifying tasks like protocol validation or path manipulation.
Conclusion
The str.partition()
method in Python is a straightforward yet powerful tool for splitting strings into a tuple based on a separator. By learning to harness this function, you enhance your ability to manage and analyze strings effectively in a variety of applications. Whether dealing with raw data, URLs, or complex structured strings, the partition()
method provides a reliable way to dissect and understand the components of your strings. Implement these techniques to maintain clean, readable, and efficient string handling in your projects.
No comments yet.