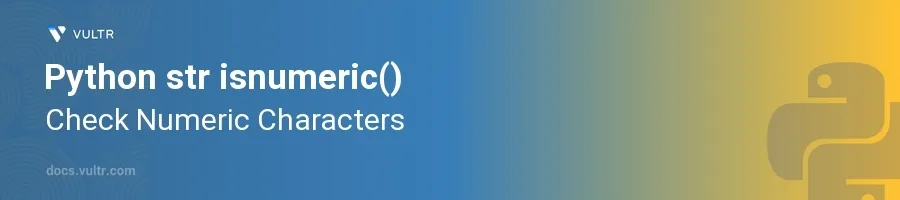
Introduction
In Python, checking if characters in a string are numeric is a common operation, especially when parsing data that could vary in format. The isnumeric()
method from the Python str
class offers a straightforward way to determine if a string contains only numeric characters. This method is especially useful in data validation, cleaning, and processing tasks where numeric input is required.
In this article, you will learn how to effectively employ the str.isnumeric()
method in various scenarios. Explore its applications in ensuring data integrity, filtering user inputs, and preparing data for numerical operations.
Understanding str.isnumeric()
Basic Usage of isnumeric()
Start by creating a simple string that contains only numeric characters.
Use
isnumeric()
to check whether the string is entirely numeric.pythonnumeric_string = "123456" result = numeric_string.isnumeric() print(result)
Here,
isnumeric()
returnsTrue
sincenumeric_string
does not contain any non-numeric characters.
Handling Non-Numeric Characters
Create a string that includes a mix of numeric and non-numeric characters.
Apply
isnumeric()
on this string to evaluate its numeric nature.pythonmixed_string = "123abc" result = mixed_string.isnumeric() print(result)
In this case,
isnumeric()
returnsFalse
becausemixed_string
includes letters, which are non-numeric characters.
Use Cases in Real-World Applications
Filtering Numeric Input from User Data
Collect input from users, such as during data entry for a form.
Use
isnumeric()
to validate that the input contains only numeric characters before processing or storing it.pythonuser_input = input("Enter numeric data: ") if user_input.isnumeric(): print("Valid numeric input received.") numeric_value = int(user_input) else: print("Non-numeric input received. Please retry.")
This snippet helps ensure that the user provides valid numeric input. If the input is purely numeric, it is converted to an integer. Otherwise, a message to retry is displayed.
Preparing Data for Mathematical Operations
Suppose you have a list of string items received from various data sources.
Filter and convert only those items that are numeric into integers for subsequent mathematical operations.
pythondata_list = ["100", "abc", "200", "-300", "400"] numeric_values = [int(item) for item in data_list if item.isnumeric()] print(numeric_values)
This code transforms strings that are numeric into integers and includes them in a new list called
numeric_values
. Non-numeric strings are excluded, ensuring reliable inputs for calculations.
Handling Unicode Characters
Understand that isnumeric()
also recognizes numeric characters from other scripts and Unicode representations, not just ASCII characters. Here’s how this works:
Utilize
isnumeric()
to check strings containing numeric characters from various global numeral systems.pythonunicode_string = "٧٨٩" # Arabic numeral characters result = unicode_string.isnumeric() print(result)
This returns
True
indicating that Arabic numerals are also recognized as numeric by theisnumeric()
method.
Conclusion
Using the str.isnumeric()
method in Python is an effective way to verify whether a string consists only of numeric characters. By incorporating this method into your data validation routines or preprocessing tasks, ensure data consistency and prevent errors in numeric computations. Whether dealing with straightforward ASCII digits or diverse Unicode numerals, isnumeric()
proves to be a reliable and indispensable tool in a programmer’s arsenal. Apply the insights and techniques explored here to maintain robust and error-free data operations in your projects.
No comments yet.