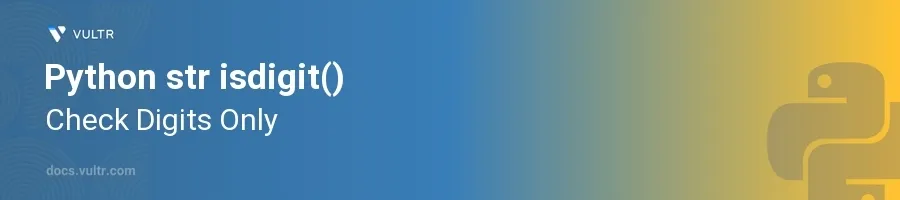
Introduction
The isdigit()
method in Python is a built-in function in the str
(string) class that checks whether all characters in a string are digits. This method is very useful when validating user input, processing data that should only contain numerical values, or performing any task that requires certainty of numeric entry in string form.
In this article, you will learn how to effectively deploy the isdigit()
method across various scenarios. Gain insights on how this method can help in data validation and understand its behavior with different types of strings, including those that may appear numeric but are not considered as such by Python.
Utilizing str.isdigit() in Data Validation
Verifying Pure Numeric Input
Begin by obtaining or defining a string that should only contain digits.
Use the
isdigit()
method to verify the content of the string.pythonuser_input = "123456" if user_input.isdigit(): print("Input is purely numeric.") else: print("Input is not purely numeric.")
This snippet checks if the
user_input
string consists only of digit characters. If all characters are digits, it confirms the input is purely numeric.
Handling Mixed Content Strings
Prepare a string that includes both digits and other characters.
Apply the
isdigit()
method to assess the string.pythonmixed_input = "123abc" result = mixed_input.isdigit() print("Is the input purely numeric? ", result)
In this example,
mixed_input
contains both digits and alphabetic characters. Theisdigit()
call returnsFalse
as not all characters are digits.
Edge Cases with Special Characters and Whitespaces
Understand that
isdigit()
returnsFalse
if there are spaces or special characters in the string.Examine the behavior of the method with a string containing spaces, punctuation, or other non-digit characters.
pythonspecial_input = "123 456" another_input = "123#456" print("Input with spaces: ", special_input.isdigit()) print("Input with special character: ", another_input.isdigit())
Here, both
special_input
andanother_input
would result inisdigit()
returningFalse
, because spaces and special characters interfere with the all-digit check.
Advanced Usage of str.isdigit()
Use in Loops for Multiple Inputs
Implement
isdigit()
in a loop to check multiple strings in sequence.Use this method to filter out non-numeric entries from a list of strings.
pythoninputs = ["123", "abc", "456", "789", "xyz"] numeric_inputs = [i for i in inputs if i.isdigit()] print("Numeric Inputs: ", numeric_inputs)
This code iterates through the
inputs
list, applyingisdigit()
to each element. It collects only those elements that are purely numeric into thenumeric_inputs
list.
Combining with Other String Methods
Couple
isdigit()
with other string methods likestrip()
to handle strings with leading or trailing spaces.Validate input effectively by cleaning up the strings before checking.
pythonspaced_input = " 2020 " cleaned_input = spaced_input.strip() print("Is cleaned input numeric? ", cleaned_input.isdigit())
The
strip()
method removes any whitespace at the beginning and end ofspaced_input
beforeisdigit()
checks if the remaining characters are all digits.
Conclusion
The isdigit()
function in Python's string class serves as a critical tool for verifying that strings contain only digit characters. Its simplicity makes it an invaluable feature in scenarios involving data validation, input verification, and the simple cleaning of numeric data from textual formats. By incorporating the isdigit()
method in your applications, you secure inputs and ensure that numerical data handled by your application is correctly formatted and error-free. Utilize this function strategically to streamline and secure data processing tasks in your Python projects.
No comments yet.