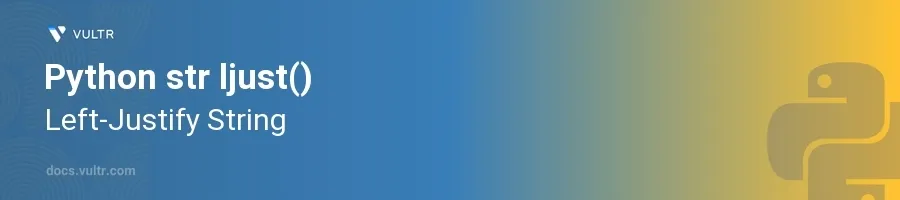
Introduction
The str.ljust()
method in Python is a straightforward yet versatile function used for string manipulation, specifically for left-justifying text within a specified width. This method ensures that all strings have a consistent width by padding them on the right side, which is particularly useful in creating well-aligned textual outputs or reformatting messages.
In this article, you will learn how to use the str.ljust()
method effectively to align strings. Understand the method's syntax, explore different scenarios such as padding with spaces or specific characters, and see how it compares to similar string functions.
Understanding str.ljust() Method
Basic Syntax and Usage
Familiarize yourself with the basic syntax of
str.ljust()
:pythonstr.ljust(width, fillchar=' ')
This method returns a new string of a given width, padding it with the
fillchar
(optional) if the original string is shorter.
Default Behavior: Padding with Spaces
Start with the simplest use case by left-justifying a string using default spaces:
pythontext = "Cat" justified_text = text.ljust(10) print("'" + justified_text + "'")
This code snippet left-justifies the string
"Cat"
inside a field of width 10. The output will be'Cat '
where spaces are used to fill the extra width.
Specifying Custom Fill Characters
Use a character other than space for padding to see a different effect:
pythontext = "Hello" justified_text = text.ljust(10, '#') print("'" + justified_text + "'")
Here, the string
"Hello"
is left-justified to the width of 10 with'#'
as the filling character. The output will be'Hello#####'
.
Practical Applications of str.ljust()
Aligning Table Columns in Text Output
Format data in tabular form using
str.ljust()
to maintain column alignment:pythontitles = ["Name", "Role", "Location"] data = [ ["Alice", "Developer", "New York"], ["Bob", "Designer", "London"], ["Catherine", "Manager", "Paris"] ] for title in titles: print(title.ljust(15), end='') print() for record in data: for item in record: print(item.ljust(15), end='') print()
Every column title and item is justified to a width of 15 characters, ensuring that all columns line up vertically, enhancing readability.
Dynamic Justification in UI Design
Adapt content dynamically for varying display sizes:
pythonuser_input = "Dynamic" screen_width = 20 justified_input = user_input.ljust(screen_width) print("'" + justified_input + "'")
A dynamic setting might involve adjusting the width based on user or display settings. The above snippet ensures the text fits perfectly across different screen widths.
Comparing str.ljust() with Other String Methods
Difference Between ljust() and rjust()
Define the use of
str.rjust()
:pythontext = "Right" print("'" + text.rjust(10) + "'")
Compare it to
ljust()
. Whileljust()
adds padding to the right,rjust()
adds padding to the left, demonstrating the utility of both depending on alignment requirements.
Combining ljust() and rjust() for Centering
Use both methods to create a centered text effect:
pythontext = "Center" left_part = text.rjust(13) full_justify = left_part.ljust(20) print("'" + full_justify + "'")
This combination of
rjust()
andljust()
effectively centers the text within the specified width, providing another way to manage text aesthetics.
Conclusion
Mastering the str.ljust()
method in Python allows you to control text layout effectively, improving both the functionality and appearance of your output. By leveraging this method to align strings left, enhance UI design, or create clean tabular outputs, you elevate the professional quality of your textual displays. Deploy this function in various applications to ensure your data is as readable and aesthetically pleasing as possible.
No comments yet.