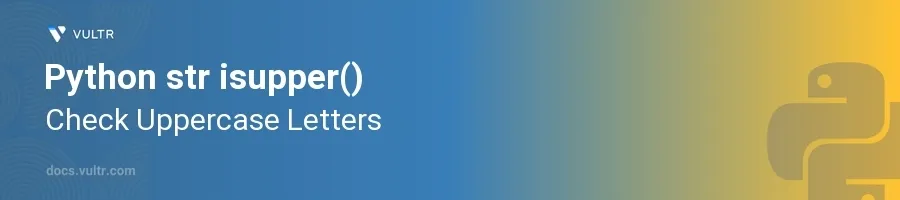
Introduction
The isupper()
method in Python is a straightforward string method used to determine if all the characters in a string are uppercase letters. This method is particularly useful when dealing with text data that requires uniformity in case, such as parsing code or validating input to ensure it conforms to specific formatting rules.
In this article, you will learn how to efficiently utilize the isupper()
method to verify the case of characters in strings. The discussion will include various examples that demonstrate the method’s utility across different scenarios, enhancing your ability to handle string manipulations in Python effectively.
Understanding the isupper() Method
The isupper()
method on a string returns True
if all the cased characters in the string are uppercase and there is at least one cased character. If the string is empty or contains no cased characters, the method returns False
.
Basic Usage of isupper()
Declare a string containing all uppercase letters.
Call the
isupper()
method on this string.pythontext = "HELLO WORLD" result = text.isupper() print(result)
Here,
text.isupper()
evaluates whether all characters intext
are uppercase. SinceHELLO WORLD
is fully uppercase, it returnsTrue
.
Testing Mixed Case Strings
Set up a string with mixed uppercase and lowercase letters.
Use
isupper()
to evaluate the string.pythonmixed_case = "Python Rules" print(mixed_case.isupper())
In this example,
mixed_case.isupper()
checks if all characters are uppercase. Since the string contains both uppercase and lowercase letters, the output will beFalse
.
Handling Non-Alphabetic Characters
Understand that non-alphabetic characters are not considered by
isupper()
.Create a string that includes digits and punctuation along with uppercase letters.
Apply
isupper()
to ascertain the case status.pythoncomplex_string = "1234!@#$%^&*()HELLO" result = complex_string.isupper() print(result)
Non-alphabetic characters, such as digits and symbols, do not influence the outcome as they are not considered 'cased' characters. Thus, since all alphabetic characters in
complex_string
are uppercase, the result isTrue
.
Practical Applications of isupper()
Understanding how to use isupper()
is one thing, but applying it in real-world situations can significantly enhance your programming efficiency. Below, you’ll find practical examples to incorporate into your projects.
Input Validation
Utilize
isupper()
to check user input for specific case requirements.Implement a function to prompt user input and validate its case.
pythondef get_uppercase_input(prompt): user_input = input(prompt) if user_input.isupper(): return user_input else: print("Please enter the text in uppercase.") return get_uppercase_input(prompt) name = get_uppercase_input("Enter your NAME in uppercase: ")
This script ensures that the user inputs their name in uppercase letters, re-prompting if the input isn't in the correct case.
Data Cleaning in Text Processing
Filter and convert strings to meet the uppercase criteria in data analysis.
Process a list of strings, converting only those that are not already uppercase.
pythontexts = ["apple", "BANANA", "Cherry"] upper_texts = [text.upper() if not text.isupper() else text for text in texts] print(upper_texts)
This snippet ensures all elements in
texts
are uppercase, promoting uniformity and potentially reducing errors in subsequent data processing tasks.
Conclusion
The isupper()
method in Python serves as a highly useful tool for string manipulation, especially when dealing with textual data that must conform to specific case requirements. Whether validating user inputs, ensuring consistency in datasets, or simply evaluating the text, isupper()
provides a straightforward solution to assess and enforce uppercase formatting. Leverage this method to keep your text data clean, consistent, and according to your programming needs.
No comments yet.