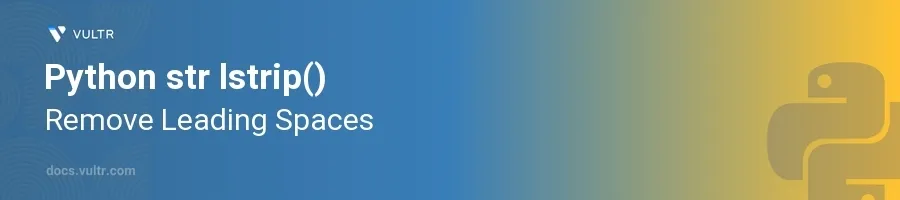
Introduction
The str.lstrip()
method in Python is a straightforward and useful string manipulation tool designed to remove leading whitespace characters from the beginning of a string. Whether the unwanted space is a space character, tab, newline, or another type of whitespace, lstrip()
handles it efficiently. This method is indispensable in data cleaning processes where precision and format consistency are crucial.
In this article, you will learn how to effectively utilize the str.lstrip()
method to clean up strings in Python. Explore how to use this method not only for removing white spaces but also for stripping specific characters from the beginning of the string. The examples will guide you through various common scenarios where str.lstrip()
proves to be beneficial.
Basic Usage of str.lstrip()
Removing Default Whitespace
Start with a string that has unwanted leading whitespace.
Apply
str.lstrip()
to remove this whitespace.pythonexample_text = " Hello, Python!" cleaned_text = example_text.lstrip() print(cleaned_text)
This code snippet removes the whitespace from the beginning of
example_text
and printsHello, Python!
. Thelstrip()
method here is used without arguments, which defaults to removing all whitespace types (spaces, tabs, newlines, etc.) at the start of the string.
Visualizing the Result
Display before and after results to affirm the effect of the
lstrip()
method.pythonprint("Original: '", example_text, "'") print("Cleaned: '", cleaned_text, "'")
This additional step helps visually confirm that the spaces preceding "Hello, Python!" have been successfully removed.
Advanced Usage of str.lstrip()
Specifying Characters to Remove
Customize which characters
str.lstrip()
should strip.Create a string prefix composed of specific characters.
pythonmessy_string = ">>>Remove this>>>Hello, Python!" cleaned_string = messy_string.lstrip('>') print(cleaned_string)
Here,
str.lstrip('>')
targets and removes leading '>' characters from the stringmessy_string
, producing the output "Remove this>>>Hello, Python!". It strips only the characters specified until it encounters a character not included in the argument.
Handle Mixed Whitespace and Characters
Understand mixing whitespaces and other characters in the
lstrip()
argument.Carefully choose the characters to be included in the removal list.
pythoncomplex_string = " ---Start Here--- Start of text" simpler_string = complex_string.lstrip(' -') print(simpler_string)
This example demonstrates the removal of spaces and dashes. The string trimming continues until it encounters a character not in the specified set (
' '
or'-'
), stopping before "Start Here--- Start of text".
Common Pitfalls in Using str.lstrip()
Avoiding Misunderstanding Strip Behavior
Be aware that
str.lstrip()
only affects the start of the string.Avoid conflicting expectations where characters in the middle or end of the string are expected to be stripped.
pythonanother_example = "Hello World " misunderstood_cleaning = another_example.lstrip() print("Unexpected outcome: '", misunderstood_cleaning, "'")
This code should clarify that
str.lstrip()
does not affect the trailing spaces at the end of the string "Hello World ". The output remains unaffected becauselstrip()
deals only with the beginning of strings.
Conclusion
Leverage the power of Python's str.lstrip()
to clean and preprocess strings effectively by removing unwanted leading characters. This method is versatile, allowing the removal of not just typical whitespaces but also bespoke sets of characters tailored to specific cleaning needs. Whether preparing data for analysis, validating user input, or simply formatting strings, str.lstrip()
offers a concise and efficient solution. As you incorporate str.lstrip()
into various tasks, enjoy cleaner, more professional, and more readable string data in your Python projects.
No comments yet.