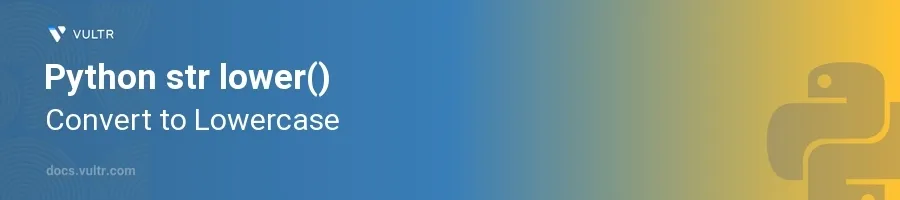
Introduction
The lower()
method in Python is an essential string operation that converts all uppercase characters in a string to lowercase. This transformation is particularly useful for standardizing text data for storage, comparison, or processing. It's often used to ensure that case differences do not affect program logic, such as during user input validation or when processing data from various sources.
In this article, you will learn how to effectively use the lower()
method on strings in Python. Explore the method's behavior with different string inputs, including mixed cases, special characters, and numbers, to understand its versatility and applications in real-world scenarios.
Implementing str lower()
Basic Usage of lower()
Start with a basic string containing mixed uppercase and lowercase characters.
Apply the
lower()
method to convert the entire string to lowercase.pythonoriginal_string = "Hello, World!" lowered_string = original_string.lower() print(lowered_string)
This code snippet converts "Hello, World!" to "hello, world!". Notice how all the uppercase letters in the original string are turned into lowercase.
Handling Strings with Numbers and Special Characters
Use a string that includes numbers and special characters along with alphabetic characters.
Demonstrate that
lower()
affects only alphabetic characters, leaving numbers and special characters unchanged.pythonmixed_string = "Python 3.8 is AWESOME! #100" lowered_mixed_string = mixed_string.lower() print(lowered_mixed_string)
In this example, only the alphabetic characters are converted to lowercase, while the numbers (
3.8
,100
) and special characters (!
,#
) remain the same.
Consistency in Data Processing
Understand the importance of consistent case for string comparisons in data processing tasks.
Apply the
lower()
method to ensure uniformity before performing comparisons.pythonuser_input = input("Enter your username: ") saved_username = "Admin" if user_input.lower() == saved_username.lower(): print("Username match!") else: print("Username does not match.")
This scenario shows a typical use case where user input is converted to lowercase to reliably compare against another string that is also brought to lowercase. This usage prevents mismatches purely due to differences in character casing.
Application in Text Normalization
Explore the application of
lower()
in natural language processing (NLP) or data pre-processing where text normalization is crucial.Normalize a list of strings to lowercase for consistent processing.
pythontitles = ["The Great Gatsby", "To Kill a Mockingbird", "1984"] normalized_titles = [title.lower() for title in titles] print(normalized_titles)
This snippet normalizes book titles to lowercase, ensuring that any subsequent text processing (like sorting or matching) is case insensitive, improving the robustness of the operations.
Conclusion
The lower()
function in Python serves as a fundamental tool for string manipulation, enabling developers to convert strings to lowercase easily and reliably. By integrating this method into string processing workflows, you ensure that your logic is less error-prone and more consistent, irrespective of how the text is originally formatted. Whether you're dealing with user inputs, normalizing data for comparison, or preparing text for natural language processing, the lower()
method streamlines your string handling tasks, making your code cleaner and more effective.
No comments yet.