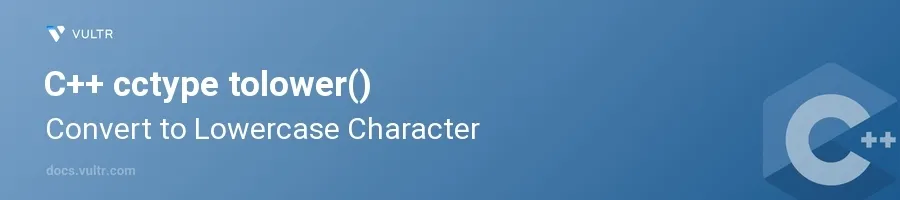
Introduction
The tolower()
function in C++ is part of the cctype
header file, providing a simple yet essential utility - converting characters to their lowercase equivalents. This function takes a single character as an input and outputs its lowercase version if it is an uppercase letter; otherwise, it returns the character unchanged.
In this article, you will learn how to effectively harness the power of the tolower()
function in various C++ programming scenarios. Explore how to transform strings and individual characters to lowercase, enhancing the uniformity and comparability of your text data.
Utilizing tolower() in C++
Convert a Single Character to Lowercase
Include the
cctype
header to accesstolower()
.Define a character variable and initialize it with an uppercase letter.
Apply the
tolower()
function and store the result.cpp#include <cctype> char upper = 'A'; char lower = tolower(upper);
This code converts the uppercase character
'A'
to its lowercase counterpart'a'
using thetolower()
function.
Transforming a Whole String to Lowercase
Include the
cctype
andiostream
headers.Initialize a string with mixed-case letters.
Iterate through each character, applying
tolower()
and construct the lowercase string.cpp#include <iostream> #include <cctype> #include <string> std::string mixedCase = "Hello World!"; std::string lowerCase; for(char c : mixedCase) { lowerCase += tolower(c); } std::cout << lowerCase << std::endl;
This snippet iterates through the string
mixedCase
, converts each character to lowercase, and assembles the results into the new stringlowerCase
. Finally, it prints"hello world!"
.
Handling Non-Alphabetic Characters
Understand that
tolower()
affects only alphabetic characters.Experiment by applying
tolower()
to a string with non-alphabetic characters to observe how these remain unchanged.cpp#include <cctype> #include <iostream> #include <string> std::string stringWithSymbols = "123 ABC!@#"; std::string result; for(char c : stringWithSymbols) { result += tolower(c); } std::cout << result << std::endl;
In this example, the digits and special symbols in the string
"123 ABC!@#"
remain unaffected while the alphabetic characters are converted to"123 abc!@#"
.
Conclusion
The tolower()
function is an essential tool in C++ for handling text transformations, particularly useful for ensuring text uniformity across different inputs that may mix uppercase and lowercase letters. By implementing the techniques discussed, you can easily convert individual characters or entire strings to lowercase, catering to various needs like case-insensitive comparisons in your applications. Use this function to maintain consistency and simplify text processing tasks in your C++ projects.
No comments yet.