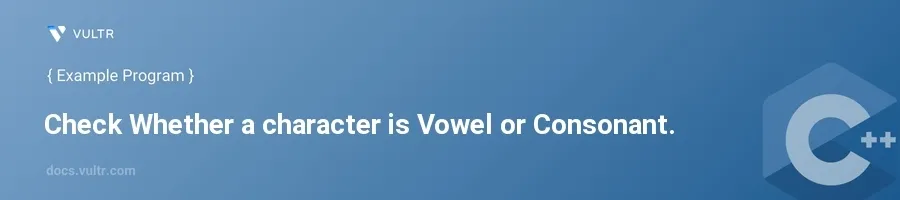
Introduction
In C++, determining whether a character is a vowel or consonant is a fundamental exercise that helps beginners understand control structures, such as conditional statements. This type of program can also introduce beginners to character handling and comparisons in C++.
In this article, you will learn how to implement a simple C++ program to check if a given character is a vowel or a consonant. You will explore examples using if-else
statements and switch-case statements, representing two different approaches to achieve the same result.
Using if-else Statement
Check Character Using if-else
Start by including the necessary header file.
Declare a character variable to store the input.
Use an
if-else
structure to determine if the character is a vowel or consonant.cpp#include <iostream> using namespace std; int main() { char c; cout << "Enter a character: "; cin >> c; if (c == 'a' || c == 'e' || c == 'i' || c == 'o' || c == 'u' || c == 'A' || c == 'E' || c == 'I' || c == 'O' || c == 'U') { cout << c << " is a vowel." << endl; } else { cout << c << " is a consonant." << endl; } return 0; }
In this code, when you provide a character, it checks against all vowels (both lowercase and uppercase). If the character matches any of the vowels, it prints that the character is a vowel; otherwise, it prints that the character is a consonant.
Using Switch-Case Statement
Check Character Using switch-case
Include the header file for input-output operations.
Initialize a character variable for the user input.
Implement a switch-case structure to handle each vowel and a default case for consonants.
cpp#include <iostream> using namespace std; int main() { char c; cout << "Enter a character: "; cin >> c; switch (tolower(c)) { // Convert character to lower case for simplicity case 'a': case 'e': case 'i': case 'o': case 'u': cout << c << " is a vowel." << endl; break; default: cout << c << " is a consonant." << endl; } return 0; }
This snippet utilizes the
switch
statement to check if a character is a vowel by converting the character to lowercase using thetolower()
function. If the character is a vowel, it is acknowledged as such; otherwise, the default case assumes it is a consonant.
Handling Non-Alphabetical Characters
Improving the Program
Incorporate a check to handle non-alphabetical inputs.
Modify the code to provide feedback if the input is not a valid letter.
cpp#include <cctype> // Include ctype.h for isalpha() function #include <iostream> using namespace std; int main() { char c; cout << "Enter a character: "; cin >> c; if (!isalpha(c)) { cout << "Please enter an alphabetic character." << endl; } else if (tolower(c) == 'a' || tolower(c) == 'e' || tolower(c) == 'i' || tolower(c) == 'o' || tolower(c) == 'u') { cout << c << " is a vowel." << endl; } else { cout << c << " is a consonant." << endl; } return 0; }
This enhanced code first checks if the character is alphabetic using the
isalpha()
function. If it isn't, it prompts the user to enter a valid alphabetic character. If the character is alphabetic, it then proceeds to check if it's a vowel or a consonant.
Conclusion
Establishing whether a character is a vowel or a consonant using C++ provides an excellent learning platform for understanding control flow in programming. Mastering the use of simple conditional statements and the switch-case structure not only helps in basic character evaluation but also sets the foundation for more complex programming tasks. Ensure to always handle possible exceptions like non-alphabetical inputs to make the program robust and user-friendly.
No comments yet.