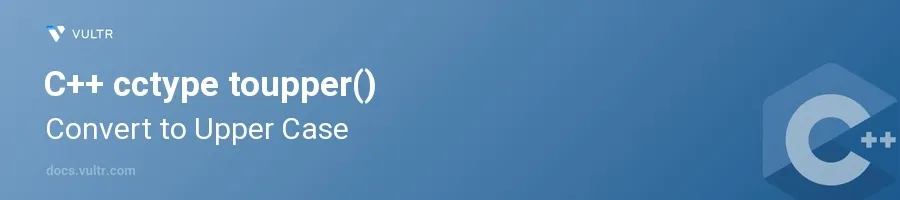
Introduction
When working with strings in C++, you might often need to manipulate their case for various reasons such as comparing, formatting, or processing user input. The toupper()
function from the C++ cctype library is tailored for such requirements, particularly for converting characters to their uppercase equivalents.
In this article, you will learn how to leverage the toupper()
function effectively. Discover applications of toupper()
to handle individual characters and strings comprehensively, and understand how to use it across different scenarios for character case manipulation.
Using toupper() with Single Characters
Convert a Single Character to Uppercase
Include the cctype header file in your C++ program.
Prepare a character variable with a lowercase letter.
Apply the
toupper()
function to this character and print the outcome.cpp#include <cctype> #include <iostream> int main() { char lowercase = 'a'; char uppercase = toupper(lowercase); std::cout << "Uppercase: " << uppercase << std::endl; return 0; }
This snippet converts the lowercase letter
'a'
to its uppercase equivalent'A'
usingtoupper()
and then outputs it.
Consider ASCII Values for Conversion
Recognize that the
toupper()
function requires the input character to be representable as an unsigned char or EOF.Ensure non-letter characters are unaffected during the conversion.
cpp#include <cctype> #include <iostream> int main() { char num = '5'; char uppercaseNum = toupper(num); std::cout << "Converted: " << uppercaseNum << std::endl; return 0; }
In this code, the numeric character
'5'
remains unchanged after passing throughtoupper()
, demonstrating that non-alphabetical characters are not converted.
Using toupper() with Strings
Convert an Entire String to Uppercase
Loop through each character in the string.
Use
toupper()
to convert each character.Store the result and display the fully converted string.
cpp#include <cctype> #include <iostream> #include <string> int main() { std::string text = "Hello, World!"; for (char &c : text) { c = toupper(c); } std::cout << "Uppercase String: " << text << std::endl; return 0; }
This code processes the string
"Hello, World!"
, converting each character to uppercase individually. The transformation is in-place, and the result is displayed as an all-uppercase string.
Conclusion
The toupper()
function in C++ provides a straightforward way to convert characters to uppercase, making it invaluable for string processing where uniform case is crucial. Whether you apply it to individual characters or iterate through strings for bulk conversion, this function ensures case manipulation is handled efficiently. Utilize these techniques in your applications to maintain consistent data presentation and simplify user input handling. With the toupper()
function, manage text data more effectively and maintain high standards of data integrity and user interaction in your C++ applications.
No comments yet.