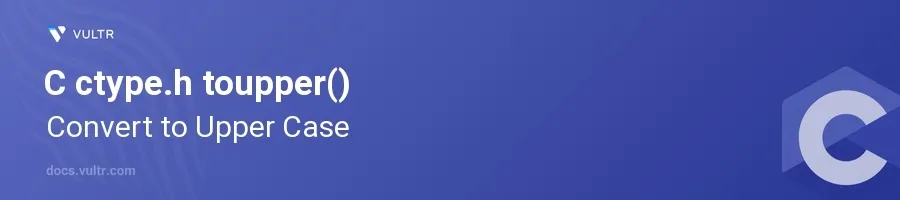
Introduction
The toupper()
function from the C standard library's ctype.h
header file is specifically designed to convert lowercase letters to uppercase. This utility is fundamental in text processing scenarios where consistent case formatting is necessary, such as data normalization processes or user input validation.
In this article, you will learn how to effectively utilize the toupper()
function in your C programs to transform lowercase characters into their uppercase counterparts. Explore both basic usage and more advanced scenarios, such as converting entire strings or handling non-alphabetic characters.
Using toupper() to Convert Single Characters
Convert a Single Character
Include the
ctype.h
library to accesstoupper()
.Define a lowercase char variable.
Apply
toupper()
and store the result.Output the converted character.
c#include <ctype.h> #include <stdio.h> int main() { char lower = 'a'; char upper = toupper(lower); printf("The uppercase version of %c is %c\n", lower, upper); return 0; }
In this code,
toupper()
converts the lowercase 'a' to its uppercase equivalent 'A'. Theprintf()
function then displays the transformation.
Handle Non-Alphabetic Characters
Understand that non-alphabetic characters remain unchanged when passed to
toupper()
.Try converting a number or special character to observe the behavior.
c#include <ctype.h> #include <stdio.h> int main() { char symbol = '@'; char result = toupper(symbol); printf("The result of converting %c is %c\n", symbol, result); return 0; }
Here, the character '@' is passed to
toupper()
. Since it's not an alphabetic character, it remains unchanged, andresult
is still '@'.
Advanced Usage of toupper()
Convert an Entire String
Recognize that
toupper()
handles one character at a time.Iterate over each character in a string, applying
toupper()
.c#include <ctype.h> #include <stdio.h> void to_upper_string(char *str) { while (*str) { *str = toupper(*str); str++; } } int main() { char str[] = "hello world!"; to_upper_string(str); printf("Uppercase string: %s\n", str); return 0; }
This example involves a function
to_upper_string
which receives a string and converts each character to upper case usingtoupper()
. Thewhile
loop continues until it reaches the end of the string, pointed by a null character.
Conclusion
The toupper()
function from ctype.h
is a versatile solution for converting lowercase letters to uppercase in the C programming language. While it primarily transforms alphabetic characters, its non-effect on non-alphabetic characters also assures that data integrity is maintained for strings with mixed content. By integrating the techniques discussed, handle text transformations with ease, ensuring that your application manages text data effectively and consistently.
No comments yet.