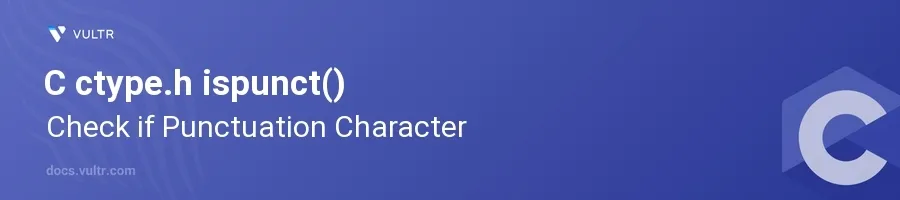
Introduction
The ispunct()
function in the C programming language is a part of the ctype.h
header file, designed to check if a given character is a punctuation mark. This utility is invaluable in situations where character validation is required, such as parser implementations or in any text processing routines.
In this article, you will learn how to effectively utilize the ispunct()
function to identify punctuation characters in input strings. This involves understanding which characters are recognized as punctuation by the function and incorporating it into real-world text processing scenarios.
Understanding ispunct()
Check if a Character is Punctuation
Include the
ctype.h
header in your C program - this contains theispunct()
function.Pass a character to
ispunct()
and check its return value.c#include <stdio.h> #include <ctype.h> int main() { char ch = '!'; if (ispunct(ch)) { printf("The character %c is a punctuation character.\n", ch); } else { printf("The character %c is not a punctuation character.\n", ch); } return 0; }
In this code snippet,
ispunct()
checks if the character stored inch
is a punctuation character. Sincech
contains'!'
, which is a punctuation mark, the function returns true and the message confirms its status.
Understanding Return Values
- Recognize that
ispunct()
returns a non-zero value (true) if the character is a punctuation mark; otherwise, it returns0
(false). - Any of the following characters are considered punctuation:
!"#$%&'()*+,-./:;<=>?@[\]^_{|}~
.
Applying ispunct() to a String
Iterate through each character in a string.
Use
ispunct()
to determine if each character is a punctuation mark.c#include <stdio.h> #include <ctype.h> void checkPunctuation(const char *str) { while (*str) { if (ispunct(*str)) { printf("'%c' is a punctuation character.\n", *str); } str++; } } int main() { const char *testString = "Hello, World! This is a test-string."; checkPunctuation(testString); return 0; }
Here, the function
checkPunctuation
receives a string and examines each character. It identifies and prints out punctuation marks found in the input stringtestString
, thus demonstrating the use ofispunct()
across an entire string.
Conclusion
The ispunct()
function from the ctype.h
library in C is highly effective for checking punctuation characters in given input. Whether you are processing a single character or an entire string, this function provides a straightforward method for punctuation validation. By incorporating ispunct()
into text processing tasks, you enhance the robustness and reliability of your data validation routines, ensuring that your programs effectively handle various kinds of input accurately.
No comments yet.