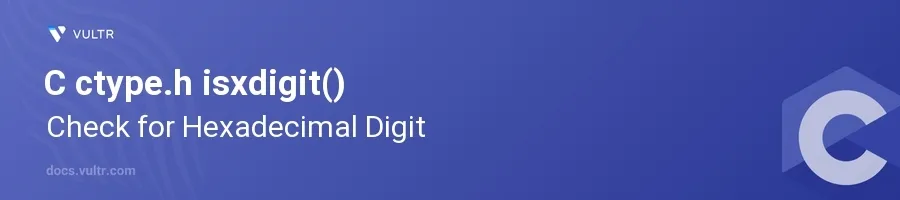
Introduction
The isxdigit()
function is an essential utility from the C ctype.h
library, primarily used to check if a given character is a hexadecimal digit. This function is invaluable when dealing with hexadecimal values, which are often used in encoding, programming involving hardware, and various high-level applications. Understanding its usage is crucial for developers working with low-level data processing or formatting.
In this article, you will learn how to efficiently employ the isxdigit()
function. Explore its functionality in validating input and parsing strings to ensure they contain valid hexadecimal characters. Additionally, delve into practical examples that demonstrate its application in everyday coding tasks.
Understanding isxdigit()
Basic Usage
Include the
ctype.h
header file in your C program to accessisxdigit()
.Pass a character to
isxdigit()
and capture its return value.c#include <ctype.h> #include <stdio.h> int main() { char ch = 'A'; int result; result = isxdigit(ch); printf("Is '%c' a hexadecimal digit? %s\n", ch, result ? "Yes" : "No"); return 0; }
This example checks whether the character 'A' is a hexadecimal digit. The
isxdigit()
function returns a non-zero value (true) if 'A' is a hexadecimal digit, and zero (false) otherwise.
Checking Strings for Hexadecimal Validity
Iterate over each character in a string.
Use
isxdigit()
to validate each character.Determine if the entire string can represent a hexadecimal number.
c#include <ctype.h> #include <stdio.h> int is_hexadecimal(const char *str) { while (*str) { if (!isxdigit(*str++)) return 0; // not a hexadecimal digit } return 1; // all characters were hexadecimal digits } int main() { const char *hex_num = "1A3F"; printf("Is '%s' a valid hexadecimal number? %s\n", hex_num, is_hexadecimal(hex_num) ? "Yes" : "No"); return 0; }
In this code, the function
is_hexadecimal
traverses a string and checks each character. If any character is not a hexadecimal digit, it returns0
. If all characters are valid, a1
is returned, confirming the string represents a hexadecimal number.
Conclusion
Utilize the isxdigit()
function from the ctype.h
library to robustly verify whether characters or strings comprise valid hexadecimal digits. This checking mechanism is particularly useful in scenarios involving data encoding, configuration settings, or anywhere hex values are predominant. Applying the insights and examples from this discussion ensures your C programs handle hexadecimal data effectively, improving both reliability and performance of data processing operations.
No comments yet.