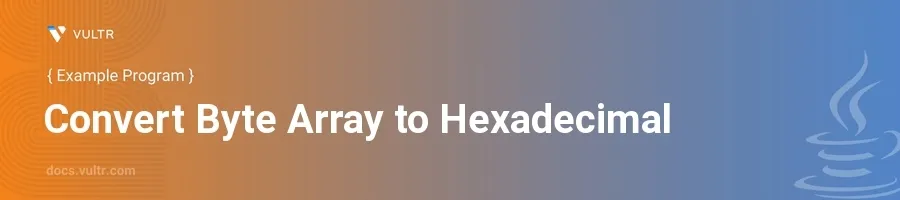
Introduction
Converting a byte array to hexadecimal in Java is a common task, especially when dealing with binary data for cryptography, networking, or file manipulations. Hexadecimal representation offers a human-readable format of binary data which is more compact compared to binary or decimal representations.
In this article, you will learn how to convert a byte array into a hexadecimal string using Java. You’ll explore several methods including manual conversion, using BigInteger
, and utilizing external libraries to achieve this. Each method is illustrated with clear examples to ensure you grasp how to implement them effectively in your Java applications.
Manual Conversion Using StringBuilder
Converting a byte array to a hexadecimal string manually involves creating a mapping between byte values and their hexadecimal counterparts. This method leverages simple string manipulation techniques.
Steps to Convert Byte Array to Hexadecimal
Create and initialize a byte array.
Prepare a
StringBuilder
to append hexadecimal values.Iterate through each byte in the array.
Convert each byte to hexadecimal using bitwise operations and append to
StringBuilder
.javapublic class ByteArrayToHex { public static String bytesToHex(byte[] bytes) { char[] hexChars = new char[bytes.length * 2]; for (int i = 0; i < bytes.length; i++) { int v = bytes[i] & 0xFF; hexChars[i * 2] = Character.forDigit((v >>> 4) & 0x0F, 16); hexChars[i * 2 + 1] = Character.forDigit(v & 0x0F, 16); } return new String(hexChars); } public static void main(String[] args) { byte[] byteArray = {10, 2, 15, 100}; String hexString = bytesToHex(byteArray); System.out.println(hexString); // Output: 0a020f64 } }
The method bytesToHex
takes a byte array and converts each byte to its hexadecimal string representation. The bitwise "AND" operation with 0xFF
preserves the byte's value as an integer. The shift and mask operations >>> 4
and & 0x0F
extract the high and low 4 bits, respectively, to correctly map them to their hexadecimal values.
Using BigInteger for Conversion
Another approach involves using Java’s BigInteger
class, which can handle large arrays of bytes and convert them into hexadecimal format effortlessly.
Steps for BigInteger Method
Create and initialize a byte array.
Use
BigInteger
to interpret the byte array as a positive number.Convert the
BigInteger
value to a hexadecimal string.javaimport java.math.BigInteger; public class ByteArrayToHexBigInteger { public static void main(String[] args) { byte[] byteArray = {10, 2, 15, 100}; String hexString = new BigInteger(1, byteArray).toString(16); System.out.println(hexString); // Output: a020f64 } }
The constructor of BigInteger
takes two parameters: a signum (1 for positive) and the byte array. The toString(16)
method converts the number to its hexadecimal string representation. Note that BigInteger
does not zero-pad, so values may not always have even length.
Utilizing External Libraries
For developers preferring to keep the codebase concise and manageable, external libraries like Apache Commons Codec and Google Guava provide utility methods for byte array conversion to hexadecimal.
Example Using Apache Commons Codec
Add the Apache Commons Codec library to your project.
Use the
Hex
class to convert the byte array into hexadecimal format.javaimport org.apache.commons.codec.binary.Hex; public class ByteArrayToHexLibrary { public static void main(String[] args) throws Exception { byte[] byteArray = {10, 2, 15, 100}; String hexString = Hex.encodeHexString(byteArray); System.out.println(hexString); // Output: 0a020f64 } }
Apache Commons Codec simplifies the conversion process to a single method call, encodeHexString
, which handles all the details internally.
Conclusion
Choose the method for converting a byte array to hexadecimal in Java that best fits your project needs. Manual conversion provides a great way to understand the underlying process and offers flexibility for customization. Using BigInteger
is efficient for handling large data sets, while external libraries offer concise and powerful solutions ideal for maintaining clean code. By integrating these methods into your Java applications, you can manage binary data more effectively and ensure your codebase remains robust and maintainable.
No comments yet.