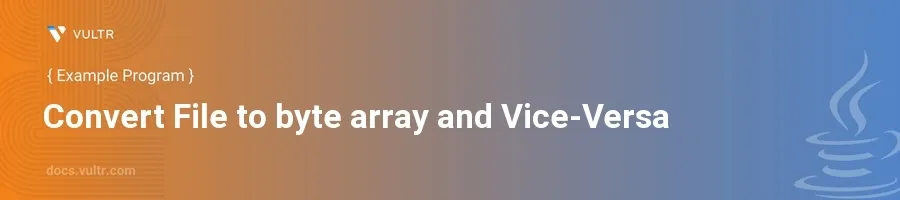
Introduction
When working with file operations in Java, converting files to byte arrays and reversing the conversion are common tasks. These operations are especially critical in situations involving file manipulation, transmission, or encryption where handling data as byte arrays can be more efficient. Byte arrays are also essential when interacting with APIs that require binary data.
In this article, you will learn how to convert files to byte arrays and then convert those byte arrays back into files using Java. This knowledge helps in scenarios like sending a file over a network where byte arrays are required or receiving a byte array to reconstruct a file.
Converting a File to a Byte Array
To convert a file to a byte array in Java, the java.io
and java.nio
packages provide comprehensive support for file operations, including reading and writing files. Here's how you handle these tasks effectively.
Read the File with FileInputStream
Import
java.io.File
andjava.io.FileInputStream
.Open a
FileInputStream
for the file you want to convert.Determine the size of the file, then read its contents into a byte array.
javaimport java.io.File; import java.io.FileInputStream; import java.io.IOException; public byte[] fileToByteArray(String filePath) { File file = new File(filePath); byte[] fileData = new byte[(int) file.length()]; try (FileInputStream fis = new FileInputStream(file)) { fis.read(fileData); } catch (IOException e) { e.printStackTrace(); } return fileData; }
In this method, create a
File
object from the specified filepath and utilizeFileInputStream
to read the file. The entire file content is read into a byte array, which is then returned.
Use Java NIO Files Class
Import
java.nio.file.Files
andjava.nio.file.Paths
.Use the
Files.readAllBytes()
method provided by NIO, which simplifies reading the file into a byte array in one line.javaimport java.io.IOException; import java.nio.file.Files; import java.nio.file.Paths; public byte[] fileToByteArrayWithNIO(String filePath) { byte[] fileData = null; try { fileData = Files.readAllBytes(Paths.get(filePath)); } catch (IOException e) { e.printStackTrace(); } return fileData; }
This method makes use of the NIO package to read all bytes from the file at once. This is generally a cleaner and more efficient way to handle file data conversion to byte arrays.
Converting a Byte Array Back to a File
Once a file is converted to a byte array, you might need to convert it back to a file format. Here's how to accomplish this using Java:
Write to File with FileOutputStream
Import
java.io.File
andjava.io.FileOutputStream
.Create a method that accepts a byte array and a file path.
Write the byte array to the file using
FileOutputStream
.javaimport java.io.File; import java.io.FileOutputStream; import java.io.IOException; public void byteArrayToFile(byte[] byteArray, String filePath) { File file = new File(filePath); try (FileOutputStream fos = new FileOutputStream(file)) { fos.write(byteArray); } catch (IOException e) { e.printStackTrace(); } }
In this approach, instantiate a
File
object and aFileOutputStream
. Pass the byte array to thewrite()
method ofFileOutputStream
, which writes it to the file system effectively.
Using Java NIO FileChannel
Import relevant NIO classes.
Create a method to write the byte array to a file using NIO
FileChannel
.javaimport java.io.IOException; import java.nio.ByteBuffer; import java.nio.channels.FileChannel; import java.nio.file.Files; import java.nio.file.Paths; import java.nio.file.StandardOpenOption; public void byteArrayToFileWithNIO(byte[] byteArray, String filePath) { try (FileChannel fileChannel = FileChannel.open(Paths.get(filePath), StandardOpenOption.CREATE, StandardOpenOption.WRITE)) { ByteBuffer buffer = ByteBuffer.wrap(byteArray); fileChannel.write(buffer); } catch (IOException e) { e.printStackTrace(); } }
This code snippet demonstrates the NIO's
FileChannel
to write a byte buffer into a file. This way provides higher performance especially for large files due to its buffer handling.
Conclusion
Converting files to byte arrays and back is a fundamental skill when managing file operations in Java. Using the methods described, streamline tasks like file transmission or storage. Adapt the techniques shown here, choosing the one best aligned with your needs, whether you prefer older java.io
classes for simplicity or the more modern java.nio
package for its performance benefits and clean code structure. By mastering these conversions, ensure your Java applications handle file data with efficiency and precision, making them robust in handling various data manipulation tasks.
No comments yet.