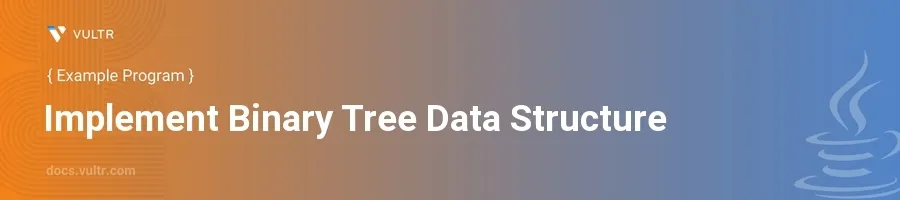
Introduction
Binary Trees are fundamental data structures in computer science which facilitate data storage and processing in a hierarchical order. Frequent applications of binary trees include database indexing, sorting algorithms, and much more, making them crucial for numerous programming and software development tasks.
In this article, you will learn how to implement a basic binary tree in Java. Explore how to initialize the tree, insert elements, and perform in-order traversal through practical examples and code snippets.
Setting up the Binary Tree Class
Define the Node Class
Start by creating the
Node
class, which will represent each element or node in the binary tree. Each node typically contains data and references to the left and right child nodes.javaclass Node { int value; Node left, right; public Node(int item) { value = item; left = right = null; } }
This class initializes the node with a value and sets both child references to null.
Create the BinaryTree Class
Define the
BinaryTree
class with an initial root node.Include methods for inserting nodes and for in-order traversal.
javaclass BinaryTree { Node root; BinaryTree() { root = null; } void insert(int value) { root = insertRec(root, value); } Node insertRec(Node root, int value) { if (root == null) { root = new Node(value); return root; } if (value < root.value) root.left = insertRec(root.left, value); else if (value > root.value) root.right = insertRec(root.right, value); return root; } }
The
insert()
method adds values by creating nodes in the correct position to maintain order. The recursive methodinsertRec()
helps in the correct placement of a new node.
Implementing Tree Traversal
In-Order Traversal
Implement a method to perform in-order traversal, which visits the left branch, then the current node, followed by the right branch. This type of traversal displays the tree's elements in their natural order.
javavoid inorder() { inorderRec(root); } void inorderRec(Node root) { if (root != null) { inorderRec(root.left); System.out.print(root.value + " "); inorderRec(root.right); } }
This recursive function traverses the tree in-order and outputs the values of the nodes in sorted order.
Example Usage
Instantiate the
BinaryTree
and populate it with values.Call the
inorder()
method to display the tree.javapublic class Main { public static void main(String[] args) { BinaryTree tree = new BinaryTree(); tree.insert(50); tree.insert(30); tree.insert(20); tree.insert(40); tree.insert(70); tree.insert(60); tree.insert(80); System.out.println("Inorder traversal of binary tree is "); tree.inorder(); } }
Running this example would print the nodes in ascending order: 20, 30, 40, 50, 60, 70, 80.
Conclusion
The binary tree is an incredibly versatile and essential data structure in Java programming, useful in various real-world applications. By constructing and traversing binary trees as demonstrated, enhance applications that require structured data storage and retrieval. Adjust and expand upon this basic structure to suit more complex needs, such as balancing the tree or implementing a binary search tree (BST) for improved performance in search operations. Implementing binary trees deeply familiarizes with concepts of recursion and object-oriented programming, paving the way for tackling more advanced data structures and algorithms.
No comments yet.