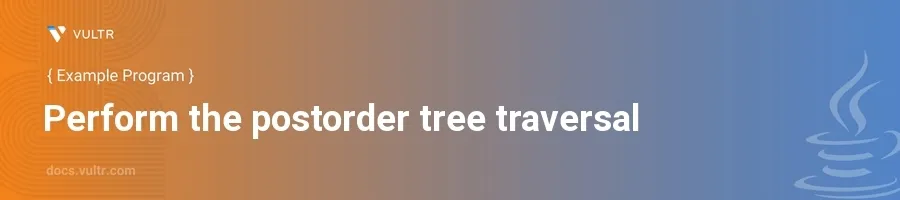
Introduction
A postorder traversal is one of the fundamental methods to visit all the nodes in a binary tree. In postorder traversal, the nodes are recursively visited in the order: left child, right child, and then the node itself. This approach is particularly useful for certain types of operations such as expression tree evaluations and file system traversals where operations on nodes are performed after their descendants.
In this article, you will learn how to implement postorder tree traversal in Java. Through examples, understand how to set up the tree structure, execute the traversal, and handle different tree configurations to enhance your understanding of tree-based algorithms.
Understanding Postorder Traversal
What is Postorder Traversal?
- Recognize that in postorder traversal, the process follows a specific node order: first visiting the left subtree, then the right subtree, and finally the node itself.
- Note that this method is used when you need to process a node only after its children nodes have been processed.
Setting up a Binary Tree Class
Define a
TreeNode
class that represents each node in the tree.Implement a constructor to initialize the node values.
javaclass TreeNode { int value; TreeNode left; TreeNode right; TreeNode(int value) { this.value = value; this.left = null; this.right = null; } }
This code snippet creates a basic structure for tree nodes with an integer value and pointers to left and right child nodes.
Creating the Tree
Instantiate the
TreeNode
class to create the nodes.Connect the nodes to form the tree as per the desired structure.
javaTreeNode root = new TreeNode(1); root.left = new TreeNode(2); root.right = new TreeNode(3); root.left.left = new TreeNode(4); root.left.right = new TreeNode(5);
In this example, a tree is constructed with the root value of
1
. The left child of the root is2
, and the right child is3
. Further, the node with value2
has two children4
(left) and5
(right).
Implementing Postorder Traversal
Recursive Approach
Define a method
postorderTraversal
to perform the traversal recursively.Implement the recursion to visit left child, right child, and then process the current node.
javavoid postorderTraversal(TreeNode node) { if (node != null) { postorderTraversal(node.left); postorderTraversal(node.right); System.out.print(node.value + " "); } }
This method first checks if the current node is not
null
. It recursively visits the left subtree, then the right subtree, and finally prints the current node’s value.
Testing the Traversal
Create a
main
method to execute the traversal.Instantiate the tree and call the
postorderTraversal
method.javapublic static void main(String[] args) { TreeNode root = new TreeNode(1); root.left = new TreeNode(2); root.right = new TreeNode(3); root.left.left = new TreeNode(4); root.left.right = new TreeNode(5); postorderTraversal(root); }
When run, this snippet outputs the values
4 5 2 3 1
, which are the nodes visited in postorder.
Conclusion
Postorder traversal is a key technique in manipulating and evaluating binary trees. By following the steps and examples provided, you can successfully implement and understand postorder tree traversal in Java. Whether managing hierarchical data or evaluating expressions, mastering this traversal method enhances your ability to work effectively with tree data structures. Adapt and apply these techniques to varied tree problems to solidify your understanding and skills in tree algorithms.
No comments yet.