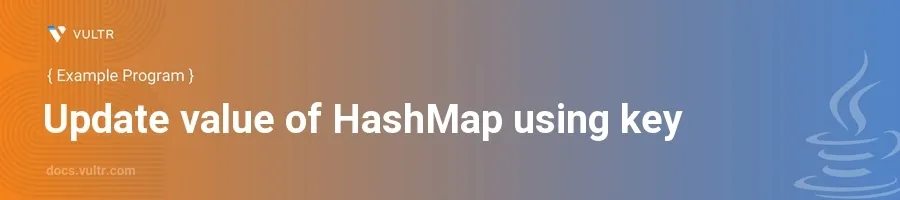
Introduction
The HashMap class in Java provides a collection for storing key-value pair mappings and is a part of the Java Collections Framework. It allows values to be stored and retrieved efficiently using keys. Manipulating these key-value pairs, especially updating the value associated with a specific key, is a fundamental operation that comes in handy in various real-world applications such as caches, database indexing, and settings configurations.
In this article, you will learn how to update the value associated with a specific key in a HashMap
. You will explore multiple examples that demonstrate this operation through simple modifications, complex data manipulation, and handling cases where keys do not already exist in the map.
Updating Values in HashMap
Modify an Existing Key's Value
Create a
HashMap
and populate it with initial key-value pairs.Update the value for a specific key using the
put
method.javaimport java.util.HashMap; public class Main { public static void main(String[] args) { HashMap<String, Integer> map = new HashMap<>(); map.put("One", 1); map.put("Two", 2); map.put("Three", 3); // Update value for key "Two" map.put("Two", 22); System.out.println("Updated HashMap: " + map); } }
The
put
method here is used to overwrite the existing value of the key "Two" from2
to22
. This method will simply replace the old value with the new one specified.
Updating Value Based on Old Value
Check if the key exists and then update its value based on the existing value.
Use the
get
method to retrieve the current value and useput
to update it.javapublic class Main { public static void main(String[] args) { HashMap<String, Double> salaryMap = new HashMap<>(); salaryMap.put("John", 30000.00); salaryMap.put("Sara", 45000.00); // Increase Sara's salary by 5000 if (salaryMap.containsKey("Sara")) { double newSalary = salaryMap.get("Sara") + 5000.00; salaryMap.put("Sara", newSalary); } System.out.println("Updated Salary Map: " + salaryMap); } }
In this code block, Sara's salary is increased by 5000. The existence of the key "Sara" in the map is checked using the
containsKey
method before updating its value to preventNullPointerException
.
Conditional Update Using compute
Method
Use the
compute
method for more complex updates, involving a remapping function.This method modifies the value of the provided key if it is present.
javaimport java.util.HashMap; public class Main { public static void main(String[] args) { HashMap<String, Integer> marks = new HashMap<>(); marks.put("Math", 85); marks.put("Science", 90); // Add extra points to Math score marks.compute("Math", (key, val) -> val == null ? null : val + 5); System.out.println("Updated Marks: " + marks); } }
Here, the
compute
method applies a lambda expression to increase the Math score by 5. This method is highly suitable for inline calculations and conditional updates.
Conclusion
Updating values in a HashMap
using their keys in Java is a straightforward yet powerful way to manipulate data within your applications. The put
method allows for simple value modifications, while methods like compute
provide flexibility for more dynamic and condition-based updates. Understanding and using these techniques ensures efficient and effective management of key-value pairs in Java, making your application's data handling both robust and adaptable. Implement these strategies in different scenarios to enhance your program's data management capabilities.
No comments yet.