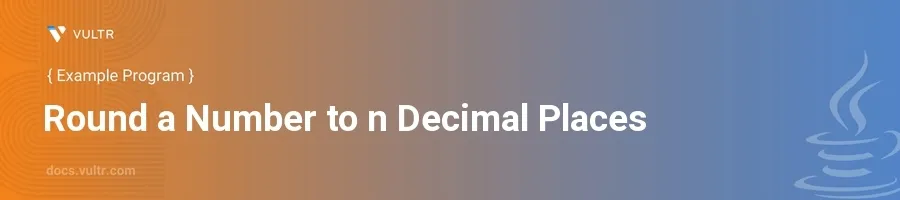
Introduction
Java, one of the most utilized programming languages, provides several methods to manipulate numerical data, including rounding numbers to a specified number of decimal places. Rounding numbers is a common requirement for applications needing precise financial calculations, statistics, or data analysis tasks where too many decimal places might complicate or slow down processes.
In this article, you will learn how to efficiently round a number to a specified number of decimal places in Java. Explore multiple methods including the use of BigDecimal
, Math.round()
, and printf()
techniques. Each method suits different scenarios and precision needs, ensuring you have the right tools for your specific tasks.
Using BigDecimal for Precise Rounding
Overview of BigDecimal
- Understand that
BigDecimal
offers unparalleled precision. BigDecimal
allows setting the scale exactly which controls the number of decimal places.
Implementing Rounding with BigDecimal
Import
BigDecimal
and related classes.javaimport java.math.BigDecimal; import java.math.RoundingMode;
This code imports the necessary classes to work with
BigDecimal
.Create a
BigDecimal
object and specify the rounding mode.javaBigDecimal bd = new BigDecimal("123.456789"); BigDecimal rounded = bd.setScale(3, RoundingMode.HALF_UP); System.out.println(rounded);
This snippet rounds the number
123.456789
to 3 decimal places using theHALF_UP
rounding mode, which is akin to traditional rounding (i.e., round half up).
Utilizing Math.round() for Basic Rounding
Overview of Math.round()
- Recognize that
Math.round()
is straightforward for quick rounding to nearest whole number. - Understand the functionality can be extended to multiple decimal places by manipulation.
Example to Round to n Decimal Places
Optionally, calculate scaling factor based on desired decimal places.
javadouble number = 123.456789; double scale = Math.pow(10, 3); // For 3 decimal places
Scaling by
10
raised to the power of the number of decimal places (here,3
).Apply
Math.round()
and adjust to the desired scale.javadouble rounded = Math.round(number * scale) / scale; System.out.println(rounded);
This example uses
Math.round()
to round the number to 3 decimal places. Thenumber
is first multiplied by1000
(scale
), rounded to the nearest whole number, and then divided by1000
.
Using printf() for Formatting Output to n Decimal Places
Overview of printf()
- Note
printf()
is primarily used for formatted output, not altering variable values. - Realize it's convenient for displaying formatted numbers without changing their storage.
Displaying Rounded Output with printf()
Use
printf()
to format and round a number during output.javadouble number = 123.456789; System.out.printf("%.3f%n", number);
This
printf()
statement formats the output ofnumber
to 3 decimal places. The format specifier%.3f
dictates this, rounding the number similarly toHALF_UP
.
Conclusion
Rounding numbers to a specific number of decimal places is a common necessity in many Java applications, and Java provides multiple ways to achieve this precision. Whether using BigDecimal
for its exactitude in financial calculations, Math.round()
for quick rounding of numbers, or printf()
for formatted outputs, Java equips you with the tools needed for effective numerical manipulation. Utilize these methods to enhance the accuracy and readability of your numerical data, ensuring results meet your precision requirements.
No comments yet.