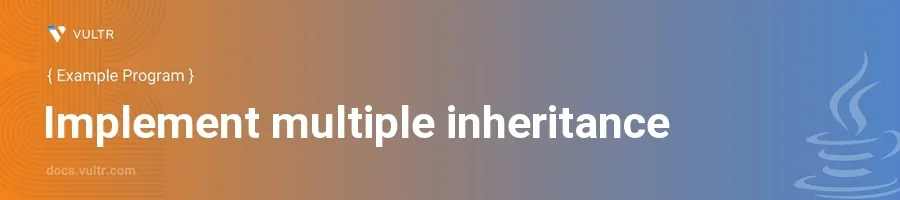
Introduction
Java, as a programming language, does not support multiple inheritance through classes due to inherent complexities and issues such as the Diamond Problem. However, Java provides a workaround to implement multiple inheritance using interfaces, which ensures that a class can inherit behavior from multiple sources. This feature is crucial for Java developers seeking to create adaptable and scalable systems.
In this article, you will learn how to effectively utilize interfaces to simulate multiple inheritance in Java. Explore through practical examples that demonstrate how a class can implement more than one interface, thereby inheriting methods from multiple sources. Each example will guide you through different scenarios using simple and engaging code snippets to illustrate the concepts clearly.
How Interfaces Simulate Multiple Inheritance
Basic Implementation of Multiple Interfaces
Define two interfaces, each with a method.
Create a class that implements both interfaces and provides concrete implementations for the interface methods.
javainterface InterfaceA { void methodA(); } interface InterfaceB { void methodB(); } class MultipleInheritanceClass implements InterfaceA, InterfaceB { public void methodA() { System.out.println("Method A from Interface A"); } public void methodB() { System.out.println("Method B from Interface B"); } }
In this example,
MultipleInheritanceClass
implements bothInterfaceA
andInterfaceB
. It provides concrete methods formethodA()
andmethodB()
which are declared in the interfaces. This demonstrates how a single class can inherit behaviors from multiple sources in Java.
Implementing Interfaces with Default Methods
Add default methods to interfaces which allows the interfaces to provide a default implementation.
Implement these interfaces in a class and override one of the default methods.
javainterface InterfaceC { default void methodC() { System.out.println("Default Method C from Interface C"); } } interface InterfaceD { default void methodD() { System.out.println("Default Method D from Interface D"); } } class EnhancedMultipleInheritanceClass implements InterfaceC, InterfaceD { public void methodD() { System.out.println("Overridden Method D in Class"); } }
Here, both
InterfaceC
andInterfaceD
provide default implementations. The classEnhancedMultipleInheritanceClass
chooses to overridemethodD()
to provide a specific implementation. This showcases an advanced use of multiple inheritance where default methods in interfaces reduce the necessity for concrete implementations in the class.
Handling Multiple Interfaces with Same Default Methods
Implement two interfaces with conflicting default methods in a single class.
Resolve the conflict by overriding the clashing method in the class.
javainterface InterfaceE { default void commonMethod() { System.out.println("Method from Interface E"); } } interface InterfaceF { default void commonMethod() { System.out.println("Method from Interface F"); } } class ConflictResolutionClass implements InterfaceE, InterfaceF { public void commonMethod() { InterfaceE.super.commonMethod(); InterfaceF.super.commonMethod(); } }
In
ConflictResolutionClass
, bothInterfaceE
andInterfaceF
have a defaultcommonMethod()
. The class must overridecommonMethod()
and decides to call both versions of the method from the respective interfaces. This clarifies how to manage method conflicts when multiple interfaces provide default implementations of the same method.
Conclusion
Interfaces in Java provide a powerful mechanism to achieve multiple inheritance while avoiding the complexity and drawbacks associated with multiple class inheritance. By utilizing interfaces, Java allows classes to inherit from multiple sources, enhancing flexibility and reusability. Through the examples provided, grasp how to use multiple interfaces effectively, deal with default method conflicts, and extend the functionality of your Java classes. Adapt these principles in your projects to harness the full potential of Java's interface-based inheritance.
No comments yet.