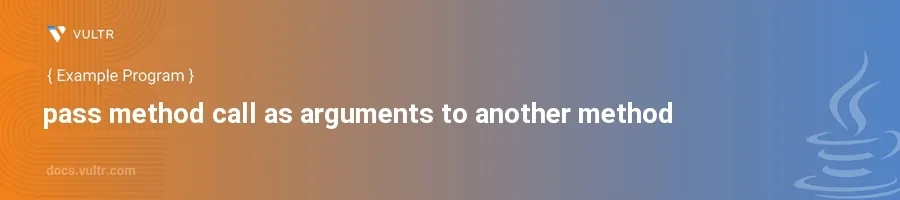
Introduction
In Java, the ability to pass method calls as arguments to another method is not as straightforward as in functional programming languages, but it can be achieved using functional interfaces and lambda expressions. This feature enhances the flexibility and reusability of your code by allowing methods to be treated as first-class citizens.
In this article, you will learn how to effectively utilize Java's capabilities to pass method calls as arguments to other methods. Explore the use of lambda expressions, method references, and functional interfaces to facilitate this, and see examples that demonstrate these techniques in action.
Understanding Functional Interfaces and Lambda Expressions
Basics of Functional Interfaces
- Grasp that a functional interface is an interface with exactly one abstract method.
- Recognize
java.util.function
package, which includes common functional interfaces likeFunction<T,R>
,Consumer<T>
, andPredicate<T>
.
Introduction to Lambda Expressions
Understand that a lambda expression provides a way to represent an instance of a functional interface using an expression.
Use lambda expressions to pass behavior as an argument.
javaFunction<String, Integer> stringLength = s -> s.length();
Here,
stringLength
is a lambda expression that implements theFunction
interface. It takes aString
and returns its length as anInteger
.
Passing Method Calls as Arguments
Using Lambda Expressions to Pass Methods
Define a method that you want to pass as an argument.
javapublic static boolean isEven(int number) { return number % 2 == 0; }
Create a method that takes a
Predicate<Integer>
as an argument.javapublic static void printFilteredNumbers(List<Integer> numbers, Predicate<Integer> predicate) { for (int number : numbers) { if (predicate.test(number)) { System.out.println(number); } } }
Use lambda to pass the
isEven
method when callingprintFilteredNumbers
.javaList<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5); printFilteredNumbers(numbers, n -> isEven(n));
Using Method References
Recognize that method references are syntactic sugar for lambda expressions where the method body directly calls another method.
javaprintFilteredNumbers(numbers, YourClass::isEven);
In this example,
YourClass::isEven
is a method reference that points to the static methodisEven
inYourClass
. It is equivalent to the lambda expressionn -> isEven(n)
.
Advanced Use Cases
Passing Instance Methods as Arguments
Understand that you can also reference instance methods.
javaclass StringProcessor { public boolean isLowerCase(String s) { return s.equals(s.toLowerCase()); } } Function<String, Boolean> checkLowerCase = new StringProcessor()::isLowerCase;
The
checkLowerCase
references the instance methodisLowerCase
.Utilize this in a similar fashion to pass method references or lambda expressions that interact with objects.
Combining with Streams API
Leverage the power of Streams and method references to process collections in a functional style.
javanumbers.stream() .filter(YourClass::isEven) .forEach(System.out::println);
This stream operation filters even numbers and prints them using method references.
Conclusion
Passing method calls as arguments in Java enhances the modularity and reusability of your code. By using lambda expressions and method references, you mirror some functional programming capabilities that allow for more expressiveness and less boilerplate code. From simple method passing to integrating this concept into Java's Streams API, the techniques discussed open up numerous possibilities for designing clean, efficient, and robust applications. Harness these strategies to make your Java programs more flexible and maintainable.
No comments yet.