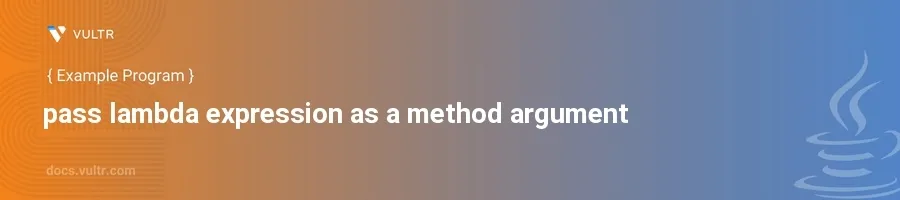
Introduction
Lambda expressions in Java provide a clear and concise way to implement single-method interfaces using an expression. These functional interfaces and lambda expressions play a significant role in handling events, carrying out operations on collections, and interacting with APIs in a streamlined manner. Essentially, they are frequently utilized to create concise code for interfaces that have only one method.
In this article, you will learn how to pass a lambda expression as an argument to a method in various scenarios. You will explore the application of lambda expressions with standard functional interfaces and custom ones. Discover these practices through practical coding examples that enhance understanding and usability in everyday programming tasks.
Using Lambda Expressions as Method Arguments
Standard Functional Interfaces
Understand that Java's Standard Library includes several functional interfaces such as
Consumer
,Supplier
,Function
, and more in thejava.util.function
package.Pass lambda expressions to manipulate or consume objects.
javaimport java.util.function.Consumer; public class LambdaExample { public static void main(String[] args) { Consumer<String> printConsumer = s -> System.out.println(s); consumeString("Hello, Lambda!", printConsumer); } static void consumeString(String str, Consumer<String> consumer) { consumer.accept(str); } }
Here, the
consumeString
method accepts aString
and aConsumer<String>
. The lambda expressions -> System.out.println(s)
is passed as a method argument, which dictates how the string is to be consumed — it simply prints the string to the console.
Custom Functional Interface
Create your functional interface that describes a targeted behavior.
Implement scenarios where passing different behaviors as lambda expressions simplifies method flexibility.
javainterface StringOperation { String operate(String s); } public class LambdaExample { public static void main(String[] args) { StringOperation toUppercase = s -> s.toUpperCase(); StringOperation appendDot = s -> s + "."; System.out.println(applyOperation("hello", toUppercase)); // Outputs "HELLO" System.out.println(applyOperation("hello", appendDot)); // Outputs "hello." } static String applyOperation(String s, StringOperation operation) { return operation.operate(s); } }
This example introduces a functional interface
StringOperation
with a methodoperate
that modifies a string. TheapplyOperation
method is designed to accept any lambda expression that matches theStringOperation
interface, demonstrating diverse utilization by converting strings to uppercase or appending a dot.
Using Lambdas with Generics
Expand lambda expression utility using generic functional interfaces to handle a variety of types.
Apply a generic method, enabling diverse type operations with a single method implementation.
javaimport java.util.function.Function; public class LambdaExample { public static void main(String[] args) { Function<Integer, Integer> squareFunction = x -> x * x; System.out.println(applyFunction(5, squareFunction)); // Outputs 25 } static <T, R> R applyFunction(T t, Function<T, R> function) { return function.apply(t); } }
In this snippet, a generic
Function<T, R>
interface is employed to create a lambda that squares an integer. TheapplyFunction
method is crafted to be universally applicable, allowing for different types of transformations based on the provided function, enhancing flexibility and reusability in various contexts.
Conclusion
Passing lambda expressions as method arguments in Java widely enhances the adaptability and succinctness of your code. By abstracting behaviors into lambdas and utilizing Java's functional interfaces, achieve concise and effective solutions to many programming tasks. From manipulating collections efficiently to custom behavior designs, these techniques ensure your methods are versatile and ready to handle diverse requirements. Implement these strategies to ensure your Java codebase remains manageable, clean, and efficient, directly benefiting from the expressiveness and power of lambda expressions.
No comments yet.