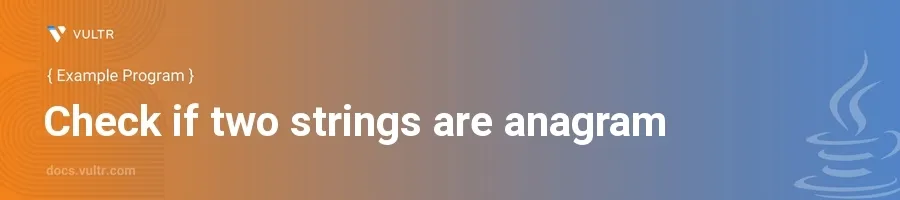
Introduction
An anagram is a word or phrase formed by rearranging the letters of a different word or phrase, typically using all the original letters exactly once. For example, the word "listen" is an anagram of "silent". In the context of programming, checking whether two strings are anagrams is a popular problem that can help understand string manipulation and algorithm implementation in Java.
In this article, you will learn how to determine if two strings are anagrams using Java. The approach will focus on examples that illustrate different methods, including sorting characters and counting frequency of characters. Each method has its own advantages and can be selected based on specific requirements of your application.
Utilizing Sorting to Check Anagrams
Simple Sorting Method
Normalize the strings by removing any spaces and converting all characters to lower case.
Convert both strings into a character array.
Sort both character arrays.
Compare the sorted arrays to check if they are identical.
javaimport java.util.Arrays; public class AnagramChecker { public static boolean areAnagrams(String str1, String str2) { char[] charArray1 = str1.replace(" ", "").toLowerCase().toCharArray(); char[] charArray2 = str2.replace(" ", "").toLowerCase().toCharArray(); Arrays.sort(charArray1); Arrays.sort(charArray2); return Arrays.equals(charArray1, charArray2); } public static void main(String[] args) { String str1 = "Listen"; String str2 = "Silent"; boolean result = areAnagrams(str1, str2); System.out.println("The two strings are anagrams: " + result); } }
This code defines a method
areAnagrams
that first normalizes the input strings by removing spaces and transforming them to lower case. It then sorts the characters of each string and checks if the sorted arrays are identical. If they are, it returnstrue
, indicating the strings are anagrams.
Using Character Frequency Count
Counting Characters with a Map
Normalize the strings by removing spaces and changing to lower case.
Use a HashMap to count the frequency of each character in the first string.
For the second string, decrease the count in the HashMap for each character.
Check if all counts in the HashMap are zero.
javaimport java.util.HashMap; public class AnagramChecker { public static boolean areAnagrams(String str1, String str2) { HashMap<Character, Integer> charCounts = new HashMap<>(); for (char c : str1.replace(" ", "").toLowerCase().toCharArray()) { charCounts.put(c, charCounts.getOrDefault(c, 0) + 1); } for (char c : str2.replace(" ", "").toLowerCase().toCharArray()) { if (!charCounts.containsKey(c)) { return false; } charCounts.put(c, charCounts.get(c) - 1); if (charCounts.get(c) == 0) { charCounts.remove(c); } } return charCounts.isEmpty(); } public static void main(String[] args) { String str1 = "Dormitory"; String str2 = "Dirty room"; boolean result = areAnagrams(str1, str2); System.out.println("The two strings are anagrams: " + result); } }
This snippet uses a
HashMap
to count the occurrences of each character in the first string and then reduces these counts based on the characters in the second string. If the map is empty at the end, then both strings had exactly the same characters with the same frequencies, confirming they are anagrams.
Conclusion
Checking if two strings are anagrams in Java can be achieved through multiple methods such as sorting the characters or counting characters using a frequency map. Each method offers a straightforward approach to solving the problem, with sorting being more concise and counting providing a more efficient solution for longer strings or when character comparison needs to be case-insensitive. By integrating these methods, you enhance the flexibility and efficiency of your string manipulation tasks in Java. Use these strategies to refine your approach to similar problems in Java programming.
No comments yet.