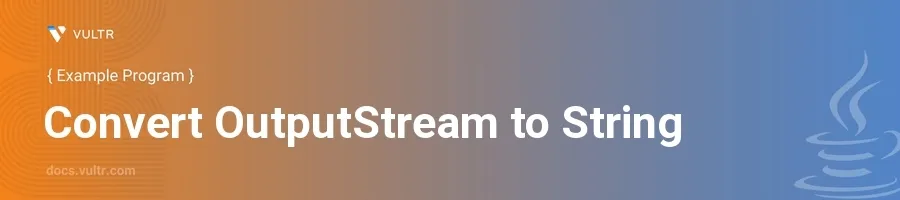
Introduction
Java provides several ways to handle and manipulate streams of data, which are a fundamental part of IO operations in the language. Converting an OutputStream
into a String
is a common task, especially when dealing with data that needs to be read back or analyzed after being written to a stream.
In this article, you will learn how to convert an OutputStream
to a String
using two distinct methods in Java. Each method will be thoroughly explained with examples that you can easily adapt to suit your project's needs.
Using ByteArrayOutputStream and toString()
Example with Basic OutputStream Operation
Begin by creating an instance of
ByteArrayOutputStream
. This class implements an output stream in which the data is written into a byte array, which can then be converted to a string.Write some data to the
ByteArrayOutputStream
.javaByteArrayOutputStream baos = new ByteArrayOutputStream(); String exampleText = "This is an example of OutputStream to String in Java."; baos.write(exampleText.getBytes());
Here, the text is converted into bytes using the
getBytes()
method and written to theByteArrayOutputStream
.Convert the content of the
ByteArrayOutputStream
to a string.javaString output = baos.toString(); System.out.println(output);
The
toString()
method ofByteArrayOutputStream
effortlessly turns the byte content into a readable string, which is then printed to the console.
Handling Character Encoding Explicitly
Understand that specifying the character encoding while converting the byte array to a string can be crucial, especially when dealing with various languages and character sets.
Write data to
ByteArrayOutputStream
as in the previous example, but convert using a specific charset when callingtoString()
.javaByteArrayOutputStream baos = new ByteArrayOutputStream(); String exampleText = "Example with UTF-8 encoding"; baos.write(exampleText.getBytes()); String output = baos.toString("UTF-8"); System.out.println(output);
Here, specifying "UTF-8" ensures that the bytes are correctly interpreted in the UTF-8 charset, making your application more robust and compatible with international use cases.
Using OutputStreamWriter and StringWriter
Direct Conversion Using StringWriter
Leverage
StringWriter
andOutputStreamWriter
to convertOutputStream
data directly into a string.First, set up your
OutputStream
connected to aStringWriter
.javaStringWriter stringWriter = new StringWriter(); try (OutputStreamWriter outputStreamWriter = new OutputStreamWriter(new WriterOutputStream(stringWriter), StandardCharsets.UTF_8)) { outputStreamWriter.write("Hello from StringWriter and OutputStreamWriter!"); } catch (IOException e) { e.printStackTrace(); } String output = stringWriter.toString(); System.out.println(output);
WriterOutputStream
adapts ajava.io.Writer
to anOutputStream
. In this case, it converts character data to byte data for theOutputStreamWriter
, which is then captured as a string by theStringWriter
. This example also handles exceptions and properly closes the stream using a try-with-resources statement.
Considerations for Charsets and Buffer Management
Master character encoding and buffer handling when using
OutputStreamWriter
for more control over data conversion.javaStringWriter stringWriter = new StringWriter(); try (OutputStreamWriter outputStreamWriter = new OutputStreamWriter(new WriterOutputStream(stringWriter), "ISO-8859-1")) { outputStreamWriter.write("Another charset example."); } catch (IOException e) { e.printStackTrace(); } String output = stringWriter.toString(); System.out.println(output);
Choosing "ISO-8859-1" as the charset handles scenarios where legacy systems or specific text data formats are involved.
Conclusion
Converting an OutputStream
to a String
in Java is essential in numerous situations, such as logging, data processing, and network communication. The methods discussed harness the power of ByteArrayOutputStream and OutputStreamWriter combined with StringWriter to achieve this conversion efficiently. Adopt these strategies to handle stream data effectively in your Java applications, ensuring data integrity and application compatibility. Explore these examples as a starting point to modify and enhance according to your specific requirements.
No comments yet.