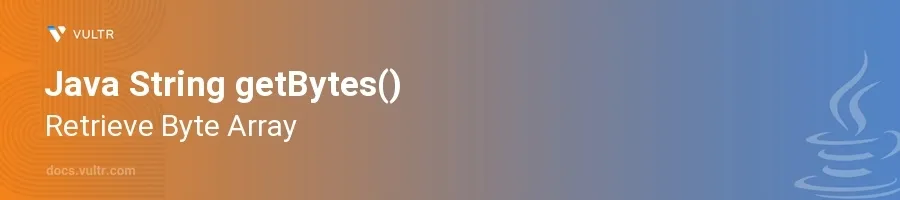
Introduction
The getBytes()
method in Java is a powerful tool for converting a String
into an array of bytes. This conversion is crucial in situations where you need to manipulate or transmit string data in its byte form, such as network communication, file handling, or when interfacing with certain APIs that require byte-level data management.
In this article, you will learn how to effectively utilize the getBytes()
method in Java. Explore how this method works with different encodings and how you can use it to transition between strings and their byte representations. Additionally, you'll understand the implications of choosing specific character sets for encoding.
Understanding the getBytes() Method
Basic Usage of getBytes()
Start with a simple string.
Use the
getBytes()
method to convert it to a byte array.Print out the byte array to see the result.
javaString example = "Hello World"; byte[] bytes = example.getBytes(); for (byte b : bytes) { System.out.print(b + " "); }
This code converts the string
"Hello World"
into its corresponding byte array. Each byte represents a character according to the system's default charset, typically UTF-8.
Analyzing Output
- Note that the output is a series of numbers. Each number corresponds to a byte in the byte array.
- Understand that in the default encoding, characters 'H', 'e', 'l', 'l', 'o', ' ', 'W', 'o', 'r', 'l', and 'd' are represented by respective bytes in the array.
Handling Non-ASCII Characters
Consider UTF-8's behavior with non-ASCII characters.
Repeat the conversion process with a string containing characters from outside the ASCII range.
javaString example = "Café"; byte[] bytes = example.getBytes(); for (byte b : bytes) { System.out.print(b + " "); }
This code snippet demonstrates how
getBytes()
handles characters like 'é' which do not fall within the standard ASCII character set.
Analyzing Output
- Expect a different output which includes negative values, indicating bytes outside the standard ASCII range.
- Recall that UTF-8 encodes characters beyond ASCII with more than one byte.
Using getBytes() with Charset
Specify Charset When Converting
Know that Java allows specifying a charset when calling
getBytes()
.Convert a string to bytes using a specific charset (e.g., US-ASCII, UTF-16).
javaString example = "Hello, World!"; byte[] utf8Bytes = example.getBytes(StandardCharsets.UTF_8); byte[] utf16Bytes = example.getBytes(StandardCharsets.UTF_16);
This example shows how to explicitly specify the UTF-8 and UTF-16 charsets for byte conversion.
Analyzing Differences in Byte Arrays
- Understand that different encodings will result in byte arrays of different lengths and values.
- Realize that UTF-16, for instance, uses more bytes for each character than UTF-8, reflecting in longer byte arrays.
Conclusion
The getBytes()
method in Java is an essential utility for converting strings into byte arrays, a common requirement in many programming scenarios ranging from file I/O to network communication. Mastery of getBytes()
not only allows handling ASCII strings but also equips to manage strings containing non-ASCII characters effectively. By understanding and applying different charsets, ensure data is accurately encoded and decoded in various applications. Utilizing the examples provided, leverage the getBytes()
method to handle string-to-byte conversions seamlessly in your Java projects.
No comments yet.