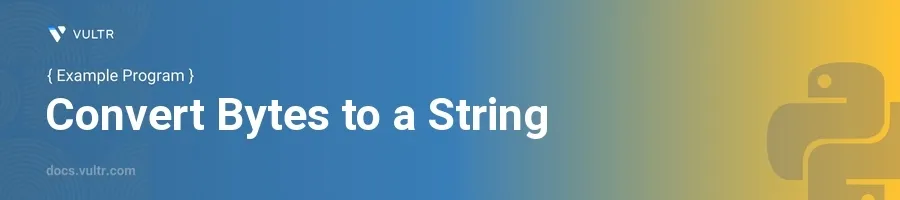
Introduction
Converting bytes to a string is a common task in Python, particularly when dealing with binary data from networks, files, or APIs. Understanding how to convert bytes into a human-readable string format is essential for data processing and manipulation in many Python applications.
In this article, you will learn how to convert bytes into strings using Python's built-in methods. Explore various examples that demonstrate effective techniques for handling different byte data and encoding types.
Basic Conversion Process
Convert Basic Bytes to String
Define a byte object.
Use the
decode()
method to convert the bytes into a string.pythonbyte_data = b'Hello, Python!' string_data = byte_data.decode('utf-8') print(string_data)
In this snippet,
byte_data
is a bytes object encoded in 'utf-8'. Thedecode()
method converts these bytes into a string. The result would display "Hello, Python!".
Handling UTF-8 Encoded Data
Include byte data with non-ASCII characters.
Decode the bytes using
utf-8
encoding to handle Unicode characters.pythonbyte_data = b'\xc2\xa9 2021 Python' string_data = byte_data.decode('utf-8') print(string_data)
This code sample involves UTF-8 bytes representing the copyright symbol followed by some text. After decoding, the output is "© 2021 Python".
Dealing with Different Encodings
Have byte data in a specific encoding other than UTF-8.
Use the
decode()
method with the appropriate encoding parameter.pythonbyte_data = b'Hola, Mundo!' string_data = byte_data.decode('latin-1') print(string_data)
Here, since the byte data is encoded in 'latin-1', specifying the correct encoding is crucial to convert the bytes correctly. The output will be "Hola, Mundo!".
Advanced Conversion Techniques
Error Handling During Decoding
Be aware that not all byte sequences can be converted into strings directly due to encoding mismatches.
Use error handling strategies like
ignore
orreplace
when decoding.pythonbyte_data = b'\xff\xfe\xfd' string_data = byte_data.decode('utf-8', errors='replace') print(string_data)
This example illustrates how to manage decoding errors by replacing bytes that cannot be decoded with a replacement character, resulting in the output "���".
Decoding from Hexadecimal Bytes
Start with hexadecimal byte data.
Convert the hexadecimal bytes to a byte string and then decode it.
pythonhex_data = '48656c6c6f' byte_data = bytes.fromhex(hex_data) string_data = byte_data.decode('utf-8') print(string_data)
This approach converts hexadecimal data representing the string "Hello" to bytes, which is then decoded back to a string.
Conclusion
Converting bytes to a string in Python is straightforward with the decode()
method, which handles various encodings and provides robust solutions for error management. Whether dealing with simple data or complex byte sequences, Python's comprehensive set of tools makes it easy to perform conversions accurately. Adopt these techniques in your Python applications for efficient data manipulation and processing.
No comments yet.