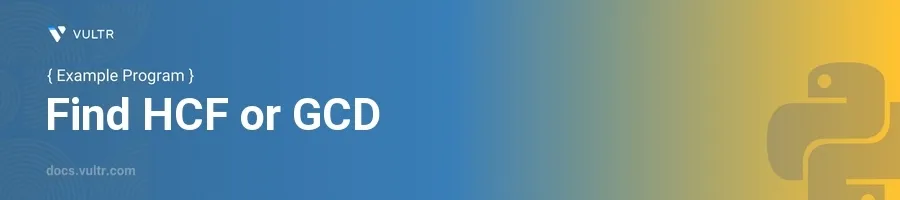
Introduction
The Highest Common Factor (HCF), also known as the Greatest Common Divisor (GCD), is an important mathematical concept commonly used to simplify fractions or find the largest common divisor of two numbers. Understanding how to compute the HCF can aid in numerous computational problems and algorithms, especially those related to number theory and cryptography.
In this article, you will learn how to calculate the HCF or GCD of two or more numbers using Python. You will explore various methods including the Euclidean algorithm, and utilize Python's built-in library to accomplish this task efficiently.
Calculate HCF with the Euclidean Algorithm
The Euclidean algorithm is a classical approach for finding the GCD of two integers. It is based on the principle that the GCD of two numbers also divides their difference.
Example Using Two Numbers
Define a function to implement the Euclidean algorithm.
Use this function to calculate the GCD of two numbers.
pythondef gcd(x, y): while y: x, y = y, x % y return x number1 = 36 number2 = 60 result = gcd(number1, number2) print("The GCD of", number1, "and", number2, "is", result)
This function takes two numbers as input and iteratively replaces one number with the remainder of these two numbers divided, until one of the numbers becomes zero. The other non-zero number at this point is the GCD.
Example Using Multiple Numbers
Extend the Euclidean algorithm to handle a list of numbers.
Apply the function to a list to find the common GCD.
pythondef gcd_multiple(numbers): result = numbers[0] for number in numbers[1:]: result = gcd(result, number) if result == 1: return 1 return result numbers_list = [24, 36, 60, 102] result = gcd_multiple(numbers_list) print("The GCD of the list is", result)
This script initializes the result with the first number in the list, then iteratively computes the GCD of the current result and each next number. This method efficiently finds the GCD for an array of numbers.
Using Python Built-ins
Python's math
module provides a built-in function gcd
which can greatly simplify the task of finding the GCD. This method is highly optimized and should be the preferred approach when working with small sets of numbers.
Use the built-in
gcd
function from Python'smath
module.Apply it directly to find the GCD of numbers.
pythonimport math number1 = 48 number2 = 180 result = math.gcd(number1, number2) print("The GCD of", number1, "and", number2, "is", result)
By importing the
math
module and using itsgcd
function, you achieve the same functionality as the earlier Euclidean method but with less code and more efficiency.
Conclusion
Determining the HCF or GCD of numbers in Python can be handled effectively using the Euclidean algorithm or Python's built-in functions. Whether you choose to implement the algorithm manually for educational purposes or utilize the built-in function for practical purposes, understanding these methods enriches your capabilities in solving problems that require fundamental number theory operations. By integrating these approaches, you ensure that your numerical computations are both robust and efficient.
No comments yet.