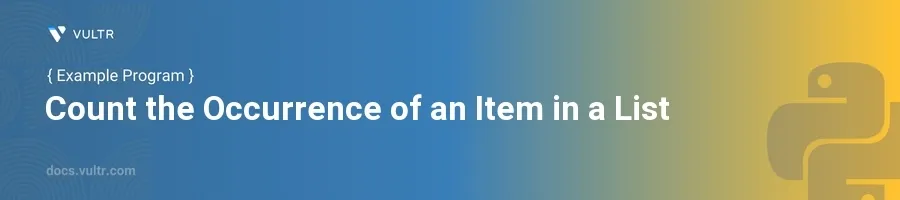
Introduction
In Python programming, counting the occurrences of an item in a list is a common operation. This can be essential for data analysis, where you need to summarize data and extract meaningful insights from lists of elements. Efficiently counting item occurrences helps in understanding the distribution of data within datasets.
In this article, you will learn how to count the number of times an item appears in a list using different methods in Python. Focus will be on using the count()
method, the collections.Counter
object, and list comprehensions with several practical examples for clarity and effectiveness.
Using the count() Method
Count Item Occurrences in a Single List
Create a list containing various elements.
Use the
count()
method to find the occurrence of a specific element.pythonitems = [1, 2, 3, 2, 4, 4, 2] count = items.count(2) print(count)
The code counts the occurrences of the element
2
in the listitems
. Thecount()
method iterates through the list and returns the number of times2
appears, which is3
in this case.
Handling Multiple Items
Handle multiple distinct elements using the
count()
method inside a loop.pythonitems = ['apple', 'banana', 'cherry', 'banana', 'cherry', 'cherry'] counts = {item: items.count(item) for item in set(items)} print(counts)
This code counts how many times each distinct fruit appears in the
items
list. Using a set for the loop helps avoid redundant counts for the same element.
Using collections.Counter
Basic Usage of Counter
Import
Counter
fromcollections
.Pass the list to
Counter
which automatically counts all items.pythonfrom collections import Counter items = ['red', 'blue', 'red', 'green', 'blue', 'blue'] counter = Counter(items) print(counter)
Counter
creates a dictionary where each key is an item from the list and its corresponding value is the count of that item. The output here shows the counts of each color.
Accessing Counts for Specific Items
Access the count for a specific item using the created counter.
pythonprint(counter['blue']) # Output the count of 'blue'
This retrieves the count of
blue
from thecounter
dictionary, which is3
.
Using List Comprehensions
Count with Conditionals
Count items meeting a specific condition using list comprehensions.
pythonitems = [1, 2, 3, 4, 5, 4, 4, 2, 1, 6, 7] count_fours = len([item for item in items if item == 4]) print(count_fours)
This code snippet counts how many times
4
appears in the listitems
. The list comprehension filters elements equal to4
, andlen()
provides the count.
Conclusion
Counting occurrences of items in a list is a fundamental task in Python that can be accomplished through various methods depending on your specific needs. Whether using the built-in count()
method for simple counts, leveraging the powerful Counter
from collections
for large datasets, or deploying list comprehensions for conditional counts, Python provides flexible, powerful tools to handle these tasks with ease. By mastering these techniques, enhance the data handling and analytical capabilities of any Python script.
No comments yet.