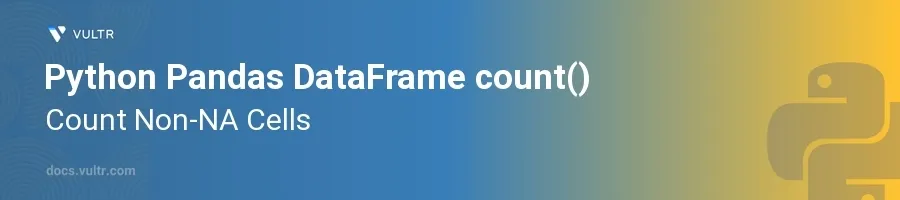
Introduction
Pandas is a prominent Python library for data manipulation and analysis, particularly well-known for its powerful data structures for handling tabular data. One such data structure is the DataFrame, which resembles a spreadsheet or SQL table and is crucial for handling large datasets efficiently. The count()
method in Pandas is specifically designed to count the non-NA/null values across the DataFrame or Series, providing essential insights into data completeness.
In this article, you will learn how to leverage the count()
function to analyze data within your DataFrame. Find out how to apply this method for single columns, multiple columns, and different axis values, thereby enhancing data understanding and preparation for further analyses. The usage of count()
will be explained through practical examples, illustrating its utility in real-world data scenarios.
Using count() to Analyze DataFrames
Counting Non-NA Cells in a Single Column
Load or create a DataFrame.
Select a particular column.
Apply the
count()
function.pythonimport pandas as pd data = {'Name': ['Alice', 'Bob', 'Charlie', None], 'Age': [25, 30, 35, 40]} df = pd.DataFrame(data) non_na_count = df['Name'].count() print(non_na_count)
Here, the DataFrame
df
includes a column "Name" with one null value. Thecount()
function counts the non-null entries, returning 3 as one entry is aNone
value.
Counting Across Multiple Columns
Load or create a DataFrame involving multiple columns.
Use the
count()
method without column specification to count non-NA cells across all columns.For column-specific counts, apply
count()
followed byaxis=0
.pythonall_columns_count = df.count() print(all_columns_count) individual_counts = df.count(axis=0) print(individual_counts)
Applying
count()
directly to the DataFramedf
returns the count of non-NA entries for each column. Specifyingaxis=0
, although redundant in this context, explicitly defines the direction along which the counts are computed, ensuring clarity.
Count Across Rows
Understand that counting across rows sums non-NA values horizontally.
Use the
axis=1
parameter with thecount()
method.pythonrow_counts = df.count(axis=1) print(row_counts)
The code counts non-null values for each row. For the DataFrame
df
, this would help understand how many attributes each entry has well-defined.
Handling Different DataTypes
Create a DataFrame with mixed datatypes.
Apply the
count()
method and observe behavior with different types.pythonmixed_data = {'id': [1, 2, 3, 4], 'score': [np.nan, 30.5, 45.2, np.nan]} df_mixed = pd.DataFrame(mixed_data) counts = df_mixed.count() print(counts)
This DataFrame
df_mixed
includesnp.nan
values which Pandas automatically treats as NA values. Thecount()
function skips these values, counting only the entries that contain data.
Conclusion
The count()
function in the Pandas library proves indispensable for exploratory data analysis, especially when preparing data for deeper analytical processes. It offers a straightforward approach to gauging dataset completeness, helping identify columns or rows with missing data, and confirming data readiness for analysis. Adapt the use of the count()
method across different axes and within subsets of your data, promoting a robust understanding and efficient handling of your datasets. By mastering these techniques, elevate the quality of data analysis tasks, ensuring that the decisions you make are data-driven and informed.
No comments yet.