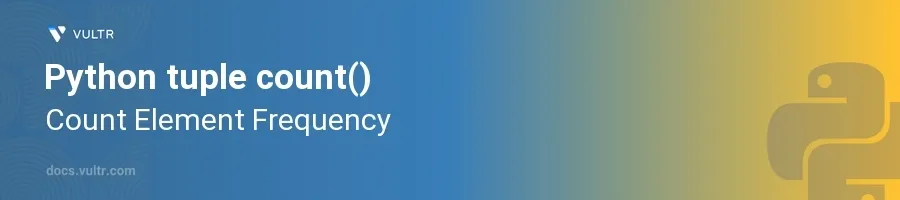
Introduction
Tuples in Python are immutable sequences of elements, used to store heterogeneous data items together. One common task when working with tuples is determining how frequently a particular element appears within them. Python provides a straightforward method, count()
, which is part of the tuple’s methods, specially designed to count the frequency of an element in a tuple.
In this article, you will learn how to accurately use the count()
method with tuples in Python. Explore various examples to understand how to apply this method in different scenarios to efficiently count occurrences of elements.
Understanding the count() Method
The count()
method is a built-in Python function available for tuple objects. It returns the number of times a specified value appears in the tuple. Knowing how to use this method effectively can be crucial for data analysis and processing tasks where you need to summarize or check data distribution.
Counting Specific Elements in a Tuple
Create a tuple with repetitive elements.
Use the
count()
method to find the frequency of a specific element.pythonexample_tuple = (1, 2, 3, 2, 1, 2, 4) count_two = example_tuple.count(2) print("Number of 2s:", count_two)
The
example_tuple
contains several instances of the integer2
. Thecount()
method computes how many times2
appears, which in this case outputs3
.
Handling Different Data Types
Recognize that tuples can contain elements of various types.
Use the
count()
method on tuples with mixed data types.pythonmixed_tuple = ('apple', 1, 'banana', 'apple', 1) count_apple = mixed_tuple.count('apple') count_one = mixed_tuple.count(1) print(f"'apple' appears: {count_apple} times") print(f"Number 1 appears: {count_one} times")
In the
mixed_tuple
, strings and integers coexist. Thecount()
function can differentiate between these types, returning2
for 'apple' and2
for the integer1
.
Case Sensitivity and Object Identity
Understand that the
count()
method differentiates based on case sensitivity and object identity.Apply this method to a tuple containing similar strings with different cases.
pythoncase_sensitive_tuple = ('Hello', 'hello', 'HELLO', 'hello') count_hello = case_sensitive_tuple.count('hello') print("Number of 'hello' (case sensitive):", count_hello)
The string 'hello' is counted specifically with the exact case used in the
count()
method, showing a count of 2 in this example. This illustrates the importance of matching cases when counting strings in a tuple.
Common Pitfalls and Tips
Awareness of some common pitfalls and tips can improve your utilization of the count()
method:
- Types Matter - Ensure that the type of the element passed to
count()
matches the type in the tuple. For example,1
and'1'
are treated differently. - Immutability - While the tuple itself is immutable, if it contains mutable items like lists, those internal items can be changed even if the tuple’s structure cannot.
- Zero Counts - If
count()
searches for an element not present in the tuple, it returns0
. Always check whether a0
return value is expected or indicates a possible error or oversight in data handling.
Conclusion
The count()
function in Python is a powerful tool for quickly assessing the frequency of specific elements within a tuple. Its straightforward application allows for efficient data analysis and error checking in tuple-based datasets. By mastering the techniques discussed, you can ensure that your data analysis and processing scripts are robust, accurate, and efficient. Remember the nuances of type and case sensitivity when using the count()
method to avoid common mistakes.
No comments yet.