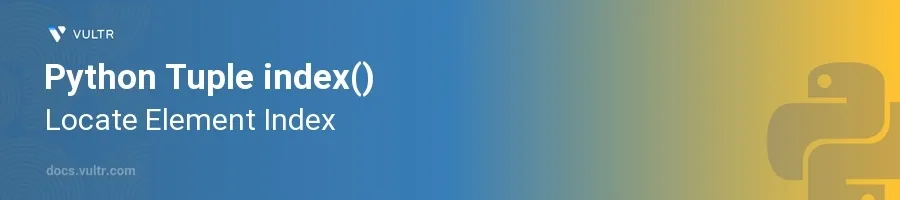
Introduction
The index()
method in Python tuples is a straightforward and efficient way to search for the first occurrence of a specified element and return its position. This method can be extremely useful when you need to pinpoint the location of particular items within a tuple, which is an immutable and ordered data structure.
In this article, you will learn how to effectively utilize the index()
method in various scenarios involving Python tuples. Explore practical examples to understand how to find the index of elements and how to handle situations when the element does not exist in the tuple.
Understanding the index() Method
Basic Usage of index()
Start with defining a tuple containing multiple elements.
Call the
index()
method by specifying the element whose index you need to find.pythonsample_tuple = (3, 6, 9, 12, 15) index_position = sample_tuple.index(9) print("The index of 9 is:", index_position)
This example demonstrates finding the index of the number
9
in the tuplesample_tuple
. Theindex()
method returns2
, since tuple indexing starts from0
.
Handling Elements Not Present in the Tuple
Understand that trying to find an index of an element not present in the tuple results in a
ValueError
.Use exception handling to deal with such cases gracefully.
pythonsample_tuple = (2, 4, 6, 8) try: index_position = sample_tuple.index(10) print("The index of 10 is:", index_position) except ValueError: print("10 is not in the tuple")
In this example, the number
10
is not a part ofsample_tuple
. Theexcept
block catches theValueError
and prints a user-friendly message.
Advanced Use Cases of index()
Finding the Index of an Element with a Start and Stop Argument
Utilize the optional
start
andstop
parameters to limit the search range within the tuple.This method helps in enhancing the search efficiency, especially in large tuples.
pythonsample_tuple = (5, 10, 15, 20, 25, 10, 30) index_position = sample_tuple.index(10, 2) print("The index of 10 after the second position is:", index_position)
The code looks for the number
10
starting from index2
ofsample_tuple
. Theindex()
method returns5
, which is the index of the second occurrence of10
.
Different Data Types Within Tuple
Understand that the
index()
method works with any data type present in a tuple.You can locate indices of strings, floating-point values, and even nested tuples.
pythonsample_tuple = ("apple", 3.142, (1, 2, 3), "banana") index_position = sample_tuple.index((1, 2, 3)) print("The index of the tuple (1, 2, 3) is:", index_position)
This snippet finds the index of a tuple
(1, 2, 3)
withinsample_tuple
. The output indicates that the nested tuple is located at index2
.
Conclusion
The index()
method in Python tuples is a valuable tool for identifying the position of elements within an immutable sequence. Whether you're working with simple or complex data structures, understanding how to use index()
effectively allows for more precise control and manipulation of tuple data. By incorporating the techniques discussed, you enhance the robustness and efficiency of your Python code. Always remember to handle exceptions appropriately to maintain the stability of your applications when elements are not found.
No comments yet.