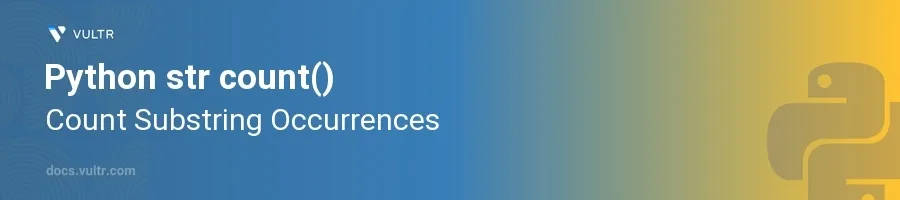
Introduction
The count()
method in Python is an integral part of string handling capabilities, allowing you to count occurrences of a substring within a string. This method helps in analyzing and manipulating textual data, whether you're dealing with user input, reading files, or pre-processing data for machine learning.
In this article, you will learn how to harness the count()
method to count substring occurrences across various scenarios. Explore practical examples to understand its usage in tackling typical string-related tasks, and become proficient in employing this method effectively.
Basic Usage of str count()
Count Direct Substring Occurrences
Start with a simple string.
Use the
count()
method to find how many times a specified substring appears.pythonmain_string = "the quick brown fox jumps over the lazy dog" count_result = main_string.count("the") print(count_result)
This code counts how many times "the" appears in
main_string
. The output will be2
because "the" appears twice.
Case-Sensitive Counting
Prepare a string with mixed case.
Apply
count()
to understand its case-sensitive behavior.pythonmixed_case_string = "Hello World, hello Python, HELLO Everyone" count_hello = mixed_case_string.count("hello") print(count_hello)
Here, since Python is case-sensitive,
count()
in this example counts only the lower-case "hello" occurrences, which appears once. TheHello
andHELLO
are not counted.
Advanced Usage of str count()
Counting Substrings in a Loop
Use
count()
inside a loop to dynamically check multiple substrings.pythonwords_to_count = ["apple", "banana", "cherry"] text = "apple banana apple cherry banana apple cherry" for word in words_to_count: print(f"Count of {word}: {text.count(word)}")
In this script, iterate over a list of words and count each one's occurrence in a given
text
. The functiontext.count(word)
is used to calculate and print each count.
Using Starting and Ending Indices
Sometimes, only a specific portion of the string needs to be examined.
Use optional parameters: the start and end indices.
pythonparagraph = "Visit the zoo. The zoo is fun. Zoo animals are cool." start_index = 12 end_index = 36 zoo_count = paragraph.count("zoo", start_index, end_index) print(zoo_count)
This code counts occurrences of "zoo" only between the indices 12 and 36. This partial section check can be impactful in large text processing, focusing analysis on relevant parts.
Using str count() with Conditions
Conditionally Count Substrings
Combine
count()
withif
statements to perform actions based on the count.pythonmessage = "remember to subscribe to the newsletter for more updates" keyword = "subscribe" if message.count(keyword) > 0: print("Keyword present!") else: print("Keyword not found.")
This snippet checks if the keyword "subscribe" appears in the message. Depending on the count, it either confirms the presence of the keyword or indicates its absence.
Conclusion
The count()
method in Python is a simple yet powerful tool for counting occurrences of substrings within strings. It provides valuable functionality for text processing, especially when paired with loops, conditions, and its optional parameters to specify segment checks. Mastering the count()
method ensures you can effectively handle and analyze strings in various Python applications, from basic scripts to complex data processing systems. By integrating the examples and techniques outlined, you'll enhance not only your string manipulation skills but also your ability to implement nuanced text analysis tasks efficiently.
No comments yet.