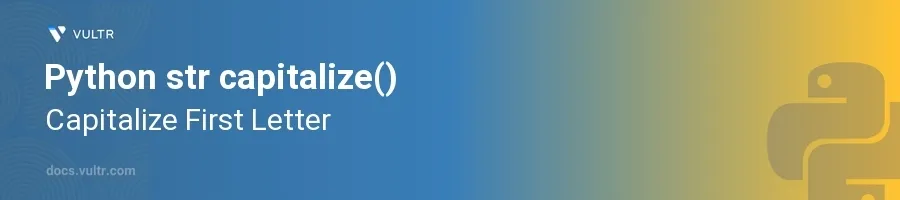
Introduction
The capitalize()
method in Python is a string operation that is quite straightforward yet essential; it transforms the first character of a string to uppercase and all other characters to lowercase. This function is widely used in formatting texts, especially in cases where proper nouns or titles need to begin with a capital letter, regardless of how they are originally entered by a user.
In this article, you will learn how to leverage the str.capitalize()
method to ensure consistent capitalization in various text processing scenarios. Grasp how to apply this method to single words, sentences, and even in more complex text manipulations involving lists and dictionaries.
Basic Usage of str.capitalize()
Capitalize a Single Word
Start with a basic string.
Apply the
capitalize()
method.pythonword = "python" capitalized_word = word.capitalize() print(capitalized_word)
The output of this code will be
Python
. Thecapitalize()
method converts the first letter of the string to uppercase and all other letters to lowercase, which is evident here since "python" becomes "Python".
Capitalizing Sentences
Consider a sentence where each word is in lowercase.
Use the
capitalize()
method to format the sentence.pythonsentence = "this is a test sentence." capitalized_sentence = sentence.capitalize() print(capitalized_sentence)
For the sentence "this is a test sentence.", the output will be "This is a test sentence.". Notice that only the first letter of the entire sentence is capitalized, while the rest remains unaffected even if they are in lowercase.
Advanced Applications of str.capitalize()
Capitalizing Each Word in a List
Compose a list of lowercase words.
Apply
capitalize()
method to each element using a list comprehension.pythonwords = ["apple", "banana", "cherry"] capitalized_words = [word.capitalize() for word in words] print(capitalized_words)
This code snippet results in the list
['Apple', 'Banana', 'Cherry']
. It demonstrates that applyingcapitalize()
in a list comprehension effectively capitalizes each word in the list individually.
Utilizing str.capitalize() in Dictionaries
Create a dictionary with strings as keys and values.
Iterate through the dictionary, capitalizing both keys and values.
pythonperson = {'first_name': 'john', 'last_name': 'doe'} capitalized_person = {key.capitalize(): value.capitalize() for key, value in person.items()} print(capitalized_person)
The dictionary
{'First_name': 'John', 'Last_name': 'Doe'}
shows that thecapitalize()
method can be applied not just to the values but also to the keys in the dictionary. This is particularly useful for improving the readability of data keys in various output formats.
Conclusion
The str.capitalize()
method in Python offers a simple yet powerful tool for string manipulation, especially useful in ensuring proper casing in text data. This method is not only limited to capitalizing single words but also extends its functionality to handling complete sentences, lists of strings, or even keys and values in dictionaries. By mastering the use of str.capitalize()
, maintain consistency and aesthetics in text outputs becomes a straightforward task. Employ this technique in your projects to enhance the professionalism and readability of textual content.
No comments yet.