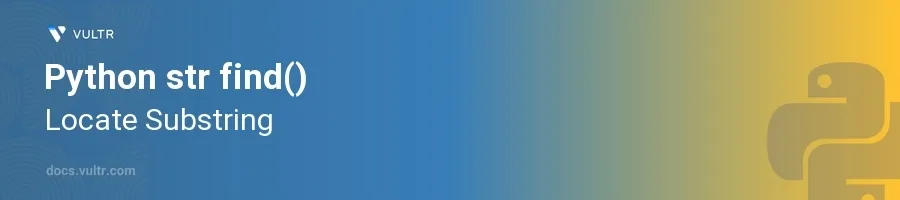
Introduction
The find()
method in Python's string class is an essential tool for locating the position of a substring within another string. It returns the index of the first occurrence of the substring. If the substring is not found, it returns -1
. This method is particularly useful in parsing text, data validation, or preprocessing steps in data analysis and machine learning pipelines.
In this article, you will learn how to effectively utilize the find()
method to locate substrings in strings. Explore practical examples that demonstrate its application in different scenarios, from basic searches to more complex string manipulation tasks.
Basic Usage of str.find()
Locate a Simple Substring
Define a string in which to search for a substring.
Use the
find()
method to locate the substring.pythonmain_string = "Hello, welcome to the world of Python." position = main_string.find("welcome") print(position)
This code checks
main_string
for the substring "welcome". As "welcome" starts at index 7, thefind()
method returns 7.
Scenario When Substring is Not Found
Search for a substring that does not exist in the main string.
Observe the returned result.
pythonmain_string = "Hello, welcome to the world of Python." position = main_string.find("goodbye") print(position)
In this example, "goodbye" is not a part of
main_string
, thusfind()
returns-1
to indicate the substring is not found.
Advanced Search with str.find()
Specify the Search Start and End Positions
Limit the search within a specific range of the main string.
Use the start and end parameters in the
find()
method.pythonmain_string = "Hello, welcome to the world of Python. Welcome again!" position = main_string.find("Welcome", 10, 50) print(position)
This snippet attempts to find "Welcome" between indices 10 and 50. The method returns the position where "Welcome" first appears in the specified range.
Case-Sensitive Search
Understand that
find()
is case-sensitive.Apply the method to find a substring matching the exact case.
pythonmain_string = "Hello, welcome to the world of Python. Welcome again!" position = main_string.find("Welcome") print(position)
find()
looks for "Welcome" with an uppercase "W". It returns the position since the case of the substring matches exactly inmain_string
.
Using str.find() in Practical Scenarios
Extracting Data Between Specific Words
Locate the start of a predefined start and end word.
Extract data between these words based on their positions.
pythonmessage = "Start here: Important data: end here." start = message.find("Start here:") + len("Start here:") end = message.find("end here.", start) data = message[start:end].strip() print(data)
Finds the substring "Important data:" by locating the indices for "Start here:" and "end here." After determining these positions, it extracts the relevant section.
Iteratively Searching for Substrings
Implement a loop to find multiple occurrences of a substring.
Continue searching from the position just after the last find until no more instances are found.
pythonmessage = "She sells sea shells on the seashore. The shells she sells are surely seashells." search_term = "shells" start = 0 positions = [] while True: start = message.find(search_term, start) if start == -1: break positions.append(start) start += len(search_term) print(positions)
This example uses a loop to find all occurrences of "shells" in the string
message
. It collects the indices of each occurrence, demonstrating how to handle multiple findings.
Conclusion
The find()
function in Python provides a compelling way to locate substrings within strings, facilitating text processing and manipulation. From finding a simple substring's occurrence to sophisticated search operations within defined ranges, find()
enhances productivity in handling string data. By mastering the examples provided, you can leverage the function's capabilities to efficiently solve problems involving text analysis and data extraction.
No comments yet.