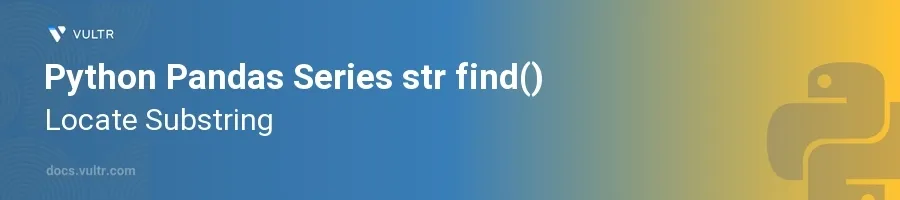
Introduction
In data manipulation and analysis, particularly with textual data, it's common to need to locate specific substrings within a larger string series. Python's pandas library offers powerful tools for dealing with such types of data efficiently, one of which is the find()
method available under the str
accessor in pandas.Series
. This method is crucial for searching the position of a substring in each element of the pandas.Series
object, enabling precise text manipulation and analysis tasks.
In this article, you will learn how to harness the find()
method within the pandas Series
object to locate substrings effectively. Explore practical examples that demonstrate searching for substrings, handling cases with missing values, and utilizing the method's parameters to refine search operations.
Basic Usage of the find() Method
Finding the Position of a Substring
Import the pandas library and create a pandas
Series
.Apply the
find()
method to search for a substring within the series.pythonimport pandas as pd data = pd.Series(['Python', 'pandas', 'Data Analysis']) position = data.str.find('a') print(position)
This code sets up a
Series
containing strings and usesfind()
to locate the first occurrence of the substring 'a'. The method returns a series with the positions of 'a' in each string or-1
if the substring is not found.
Handling Case Sensitivity
Implement the find() method to demonstrate its case sensitivity.
Use the
lower()
function to ensure case-insensitive matching.pythondata = pd.Series(['Python', 'pandas', 'Data Analysis']) position_case_sensitive = data.str.find('P') position_case_insensitive = data.str.lower().find('p') print("Case Sensitive Positions:\n", position_case_sensitive) print("Case Insensitive Positions:\n", position_case_insensitive)
This snippet first checks for 'P' considering the case, then it transforms each element in the series to lowercase and searches for 'p', ensuring that the search is case-insensitive.
Advanced Search Parameters
Specifying Start and End Parameters
Utilize the
start
andend
parameters to define the search area within the strings.Observe how these parameters influence the outcome of the
find()
method.pythondata = pd.Series(['Python programming', 'pandas library', 'Data Analysis']) position_limited_search = data.str.find('a', start=1, end=10) print(position_limited_search)
By specifying start and end parameters, you limit the search for 'a' between the 1st and 10th characters of each string. This provides more control over where the search occurs.
Working with Missing Data
Address missing values appropriately when using the
find()
method.Check for missing values in the series to avoid errors during substring finding.
pythondata = pd.Series(['Python', None, 'Data Analysis']) position_with_na = data.str.find('a') print(position_with_na)
This example shows that
find()
handles missing values (None
) gracefully by returningNaN
where applicable, ensuring the integrity of your data analysis process.
Conclusion
The str.find()
method in Python's pandas library is an essential tool for locating the position of substrings within a Series
. Accurate and efficient, it allows for robust textual data manipulation, including handling variations in case sensitivity and processing within defined limits of the strings. By mastering the use of the find()
method, as demonstrated in the practical examples above, you elevate your data analysis capabilities, effectively handling both straightforward and complex text searching tasks in your datasets.
No comments yet.