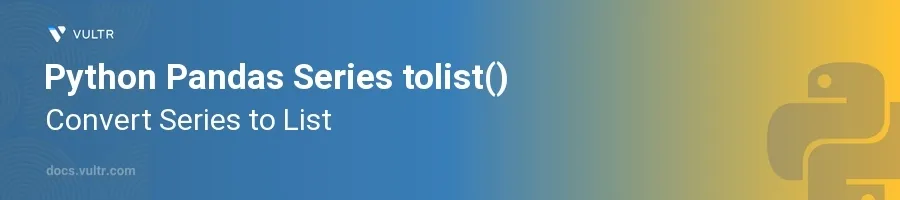
Introduction
Pandas is a cornerstone tool for data manipulation and analysis in Python, primarily used to manipulate large datasets efficiently and easily. A frequent requirement when working with Pandas is transferring data from a Series
object to a standard Python list. This is where the tolist()
method comes into play, serving as a bridge between Pandas’ powerful data handling capabilities and Python’s versatile list.
In this article, you will learn how to utilize the tolist()
method to convert a Pandas Series into a Python list. Explore practical examples to understand how to apply this method effectively in your data processing tasks, ensuring seamless data manipulation and preparation for further analysis or visualization.
Understanding Series and tolist() Method
What is a Pandas Series?
- Recognize a Pandas Series as a one-dimensional array capable of holding any data type.
- Note that each element in a Series has an associated label, known as an index.
How tolist() Method Works
- Acknowledge that the
tolist()
method converts a Pandas Series into a regular Python list. - Understand that the indices of the Series are not included in the list; only the values are converted and stored.
Converting Series to List
Basic Conversion of a Series to a List
Import the Pandas library and create a simple Series.
Use the
tolist()
method to convert the Series to a list.pythonimport pandas as pd # Creating a Series data_series = pd.Series([1, 2, 3, 4, 5]) # Converting Series to List data_list = data_series.tolist() print(data_list)
This code creates a Series with integers and converts it to a list. The output will be
[1, 2, 3, 4, 5]
.
Working with Different Data Types
Create a Series with mixed data types.
Convert the mixed data type Series to a list and observe the output.
pythonmixed_series = pd.Series([10, 'Pandas', 3.14, True]) # Converting to List mixed_list = mixed_series.tolist() print(mixed_list)
Here, the Series containing an integer, string, float, and boolean converts seamlessly into a Python list.
Converting a Series with Index to a List
Create a Series with a custom index.
Convert the Series to a list, showing only values are included.
pythonindexed_series = pd.Series([10, 20, 30, 40], index=['a', 'b', 'c', 'd']) # Converting to List indexed_list = indexed_series.tolist() print(indexed_list)
Despite the custom indices, the
tolist()
function only extracts the values[10, 20, 30, 40]
, disregarding the indices.
Practical Applications and Considerations
When to Use tolist() in Data Analysis
- Convert a Series to a list for use in functions or operations that require a list input.
- Transform Series data to list before exporting or integrating with other Python modules that do not support Pandas Series.
Data Integrity and Transformation
- Verify that all data types convert correctly and no data is unexpectedly altered during the conversion.
- Be cautious that converting large series can be memory-intensive as lists do not handle large data as efficiently as Series.
Limitations and Alternatives
- Acknowledge the loss of index information in the conversion process—consider alternatives if index preservation is necessary.
- Consider using
numpy
arrays which are more memory efficient for large datasets.
Conclusion
Using the tolist()
method to convert a Pandas Series into a Python list is straightforward and efficient, catering well to diverse data types and structures. This method becomes crucial when interfacing Pandas with functionalities that operate primarily on lists. Adapt the use of tolist()
in various scenarios discussed, and remember its limitations to make informed choices regarding data manipulation in Python. With these techniques, you ensure the swift and effective handling of data conversion, paving the way for broader application scope in programming projects.
No comments yet.