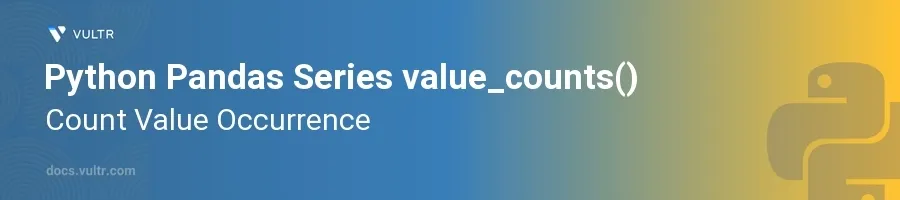
Introduction
The value_counts()
method in the Pandas library is invaluable when dealing with data analysis, particularly when you need to count the occurrence of each unique value in a series. This method helps in summarizing and visualizing data, allowing analysts to quickly understand the distribution of data across various categories.
In this article, you will learn how to use the value_counts()
method in different scenarios. Discover how to perform simple value counts, handle missing values, normalize the results, and even segment the counts by categories. These skills will empower you to effectively handle and analyze datasets in Python using Pandas.
Basic Usage of value_counts()
Counting Unique Values in a Series
Import the Pandas library and create a simple Pandas Series.
Apply the
value_counts()
method to count the occurrences of each unique value.pythonimport pandas as pd data = pd.Series([1, 2, 2, 3, 3, 3, 4, 4, 4, 4]) value_counts = data.value_counts() print(value_counts)
This code snippet creates a series from a list of integers. Using
value_counts()
generates a new series where the index represents the unique values from the original series, and the values are the counts of each unique entry.
Handling Missing Data
Include NaN values in your series data.
Utilize the
dropna
parameter invalue_counts()
to include or exclude NaN values in the count.pythonimport numpy as np data = pd.Series([1, 2, np.nan, 2, 3, np.nan]) value_counts = data.value_counts(dropna=False) print(value_counts)
In this example, the series includes NaN (missing) values. By setting
dropna=False
,value_counts()
includes NaN in the output, helping in a complete assessment of data availability.
Advanced Options in value_counts()
Normalizing the Results
Normalize the results to get the relative frequencies of the values.
Apply the
normalize=True
parameter in thevalue_counts()
method.pythondata = pd.Series([1, 2, 2, 3, 3, 3]) normalized_counts = data.value_counts(normalize=True) print(normalized_counts)
This snippet normalizes the count results to show the proportion of each unique value relative to the total number of occurrences, making it easier to understand the distribution of data.
Sorting the Results
Control the sorting of the counts.
Use the
sort
andascending
parameters to manage the order of the result set.pythondata = pd.Series([1, 2, 2, 3, 3, 3, 4, 4, 4, 4]) sorted_counts = data.value_counts(sort=True, ascending=False) print(sorted_counts)
By default,
value_counts()
sorts the counts in the descending order of occurrence. You can adjust this behavior using thesort
andascending
parameters as demonstrated.
Excluding Less Frequent Values
Filter out values below a certain threshold of occurrence.
Use boolean indexing with the results from
value_counts()
.pythondata = pd.Series([1, 2, 2, 3, 3, 3, 4, 4, 4, 4, 5]) value_counts = data.value_counts() filtered_counts = value_counts[value_counts > 2] print(filtered_counts)
This example first calculates the value counts. Then, it uses boolean indexing to keep only those values that occur more than twice.
Conclusion
Master the value_counts()
method in Pandas to vastly improve data handling and analysis processes. With the ability to count occurrences, normalize results, and manage counting of NaN values, your data analysis becomes more straightforward and insightful. Utilize these counting techniques to aid in everything from preliminary data exploration to deep data analysis, ensuring your datasets are well-understood and effectively used. Use the examples and techniques discussed as a foundation for adapting the value_counts()
method to specific data analysis needs.
No comments yet.