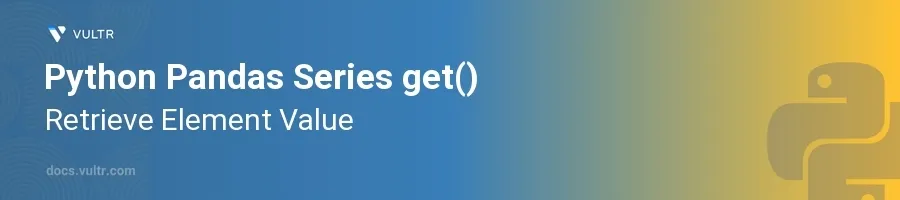
Introduction
The get()
method in Python's Pandas library is a versatile tool for retrieving elements from a Series object using index labels. This method is crucial when working with datasets as it provides a safe way to access data, avoiding the risk of raising errors when querying non-existent keys. This characteristic makes get()
particularly useful in data analysis where missing values are common.
In this article, you will learn how to effectively utilize the get()
method in various scenarios to retrieve values from a Series. The focus is on showcasing how to access data safely, handle missing keys gracefully, and utilize additional parameters to enhance data retrieval processes.
Exploring Basics of get()
Retrieve a Simple Value Safely
Start with creating a simple Pandas Series.
Use the
get()
method to fetch an element by its index.pythonimport pandas as pd data = pd.Series([10, 20, 30], index=['a', 'b', 'c']) result = data.get('b') print(result)
This example demonstrates retrieving the value
20
from the seriesdata
where the index is 'b'. Theget()
method returns the value without raising an exception even if the index is not found.
Handling Non-existent Keys
Use the
get()
method to attempt retrieving a value with a non-existent key.Observe the response when the key is missing.
pythonresult = data.get('x') print(result)
Unlike direct indexing which would raise an error if the key does not exist,
get()
returnsNone
. This feature is particularly useful in data processing tasks where the presence of a key cannot be guaranteed.
Specifying a Default Value
Use the
get()
method with a default value to handle missing keys gracefully.Set a custom return value for cases where the requested index is missing.
pythondefault_value = 0 result = data.get('x', default_value) print(result)
Here, instead of receiving
None
for a non-existent key,get()
returns the specifieddefault_value
, which is0
. This technique allows for more controlled error handling and data manipulation.
Advanced Usage of get()
Retrieving Values from a Series with a Complex Index
Create a Pandas Series with a more complex index structure like a MultiIndex.
Fetch data using
get()
from the Series with a tuple representing the MultiIndex key.pythonindex = pd.MultiIndex.from_tuples([('a', 1), ('b', 2), ('c', 3)]) data = pd.Series([100, 200, 300], index=index) result = data.get(('b', 2)) print(result)
The output here will be
200
. This example illustrates howget()
can seamlessly handle complex indexing, making it a valuable method for multi-dimensional data structures.
Using get() in Conditional Expressions
Integrate the
get()
method in conditional statements to check for the existence of keys.Perform conditional operations based on the return value of
get()
.pythonif data.get('a', None) is not None: print("Key exists") else: print("Key does not exist")
This usage demonstrates how
get()
can be pivotal in conditional logic workflows where decisions depend on the presence or absence of specific data elements.
Conclusion
The get()
method in Pandas is a powerful function for safely accessing data within a Series. Its ability to handle missing keys by returning None
or a specified default value makes it ideal for robust data processing tasks. By incorporating the techniques discussed, such as handling complex indexes and integrating get()
into conditional statements, you ensure your data access patterns are not only effective but also resilient to issues arising from non-existent data points. Use this tool to simplify data extraction and enhance the manageability of your data analysis projects.
No comments yet.