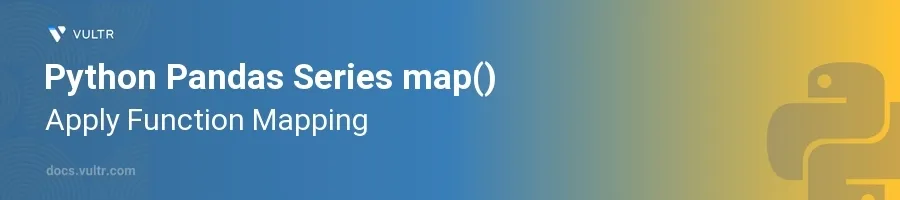
Introduction
In many data manipulation tasks, especially in data science and analytics, you might need to apply transformations to data elements in an efficient and concise manner. The map()
function in the Pandas Series object is a powerful tool designed for this purpose. It allows you to map an existing value of a Series to a different set using a dictionary or function, which can be extremely beneficial for data preprocessing and transformation.
In this article, you will learn how to effectively use the map()
function in various scenarios using Python's Pandas library. Explore how to apply simple transformations, handle missing data, and enhance performance in your data manipulation tasks.
Understanding the Basics of Series.map()
The map()
function is applicable specifically to Pandas Series and can be used to replace or transform each element in the series with another value. The transformation or replacement can be defined using a function, a dictionary, or a Series.
Apply Simple Function Mapping
Start with importing the Pandas library and creating a simple Pandas Series.
Define a function that you intend to apply to each element.
Use the
map()
function to apply this transformation.pythonimport pandas as pd # Creating a Pandas Series series_data = pd.Series([1, 2, 3, 4, 5]) # Function to square each element def square(x): return x ** 2 # Applying function using map() squared_data = series_data.map(square) print(squared_data)
This code snippet defines a function
square()
that squares a number. Themap()
function is then used to apply this function across all elements ofseries_data
, resulting in a new Series of squared values.
Mapping with a Dictionary
Create a Pandas Series with elements you need to transform based on certain conditions or categories.
Define a dictionary where keys represent the existing elements, and the values represent the new values after mapping.
Pass the dictionary to the
map()
function to perform the mapping.python# Series with categorical data series_cats = pd.Series(['cat', 'dog', 'bird', 'dog', 'cat']) # Dictionary to map categories to numbers category_map = {'cat': 1, 'dog': 2, 'bird': 3} # Mapping categories to numbers mapped_cats = series_cats.map(category_map) print(mapped_cats)
Here, every instance of 'cat', 'dog', and 'bird' in
series_cats
is replaced with 1, 2, and 3, respectively, using thecategory_map
dictionary.
Handling Missing Values in map()
Prepare a Pandas Series which includes some missing values.
Utilize a dictionary to map existing values to new values, ensuring the dictionary handles scenarios universally (either by including a default or by excluding unmatched items).
Use
map()
and observe the treatment of missing or unmatched items.python# Series with missing values series_missing = pd.Series(['apple', 'banana', 'carrot', None]) # Mapping only certain items fruit_map = {'apple': 'fruit', 'banana': 'fruit'} # Applying map mapped_fruits = series_missing.map(fruit_map) print(mapped_fruits)
In this example, 'apple' and 'banana' are mapped to 'fruit', while 'carrot' and
None
are replaced withNaN
since they are not found in thefruit_map
dictionary.
Mapping Using Another Series
Sometimes, it becomes necessary to use another Series as a mapping reference, whereby the index in one series aligns with values in another series.
Create two Series, one as a mapper and another containing values to be mapped.
Make sure the indices of the mapper Series correspond to the values of the input Series.
Use the
map()
function by passing the mapper Series.python# Target Series and a Mapper Series input_series = pd.Series([0, 1, 2]) mapper_series = pd.Series(['zero', 'one', 'two']) # Using mapper Series result = input_series.map(mapper_series) print(result)
Here, the numbers 0, 1, and 2 in
input_series
are replaced with 'zero', 'one', and 'two', usingmapper_series
where indices directly align with the values.
Conclusion
The map()
function in Pandas is a versatile tool for data transformation, offering various ways to apply complex mappings and transformations with minimal code. It supports using functions, dictionaries, or even other Series to define the mapping logic, providing a high level of flexibility and power in data preprocessing workflows. By mastering the map()
function, you enhance your ability to perform efficient and effective data manipulations, leading to cleaner, more readable, and more efficient data science workflows.
No comments yet.